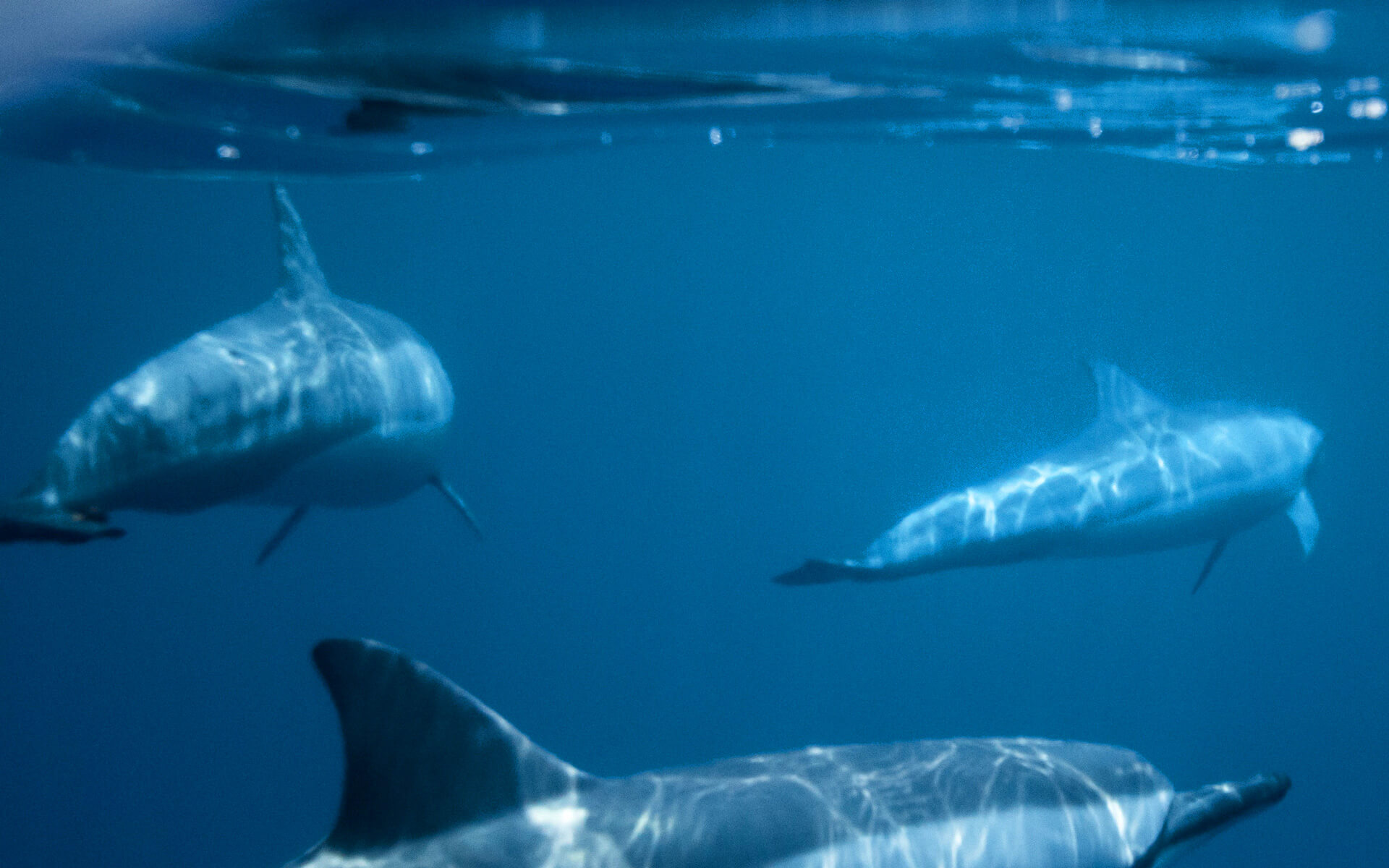
Getting Django To Talk To MySQL 8
November 19, 2019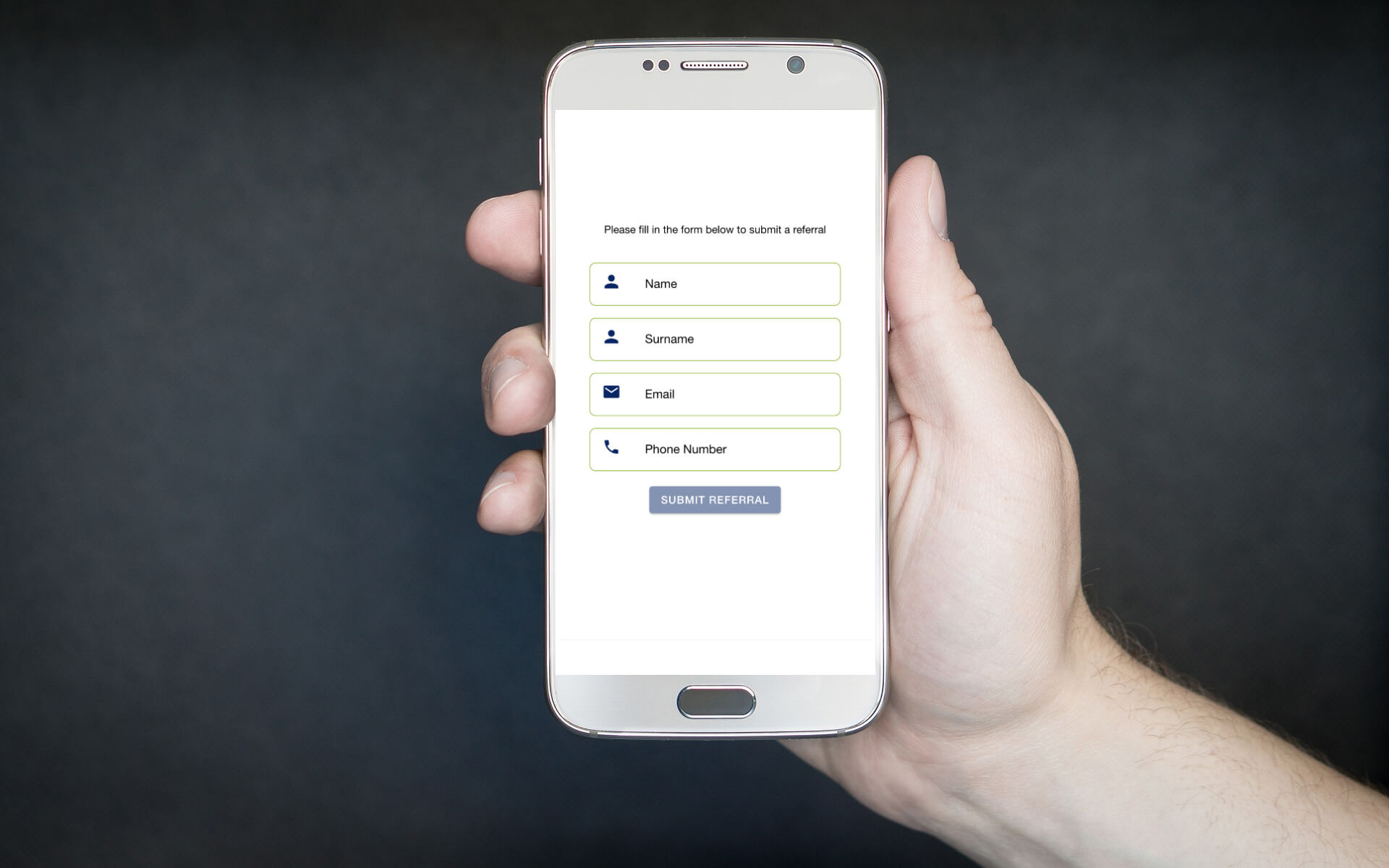
Ionic Custom Form Builder
January 6, 2020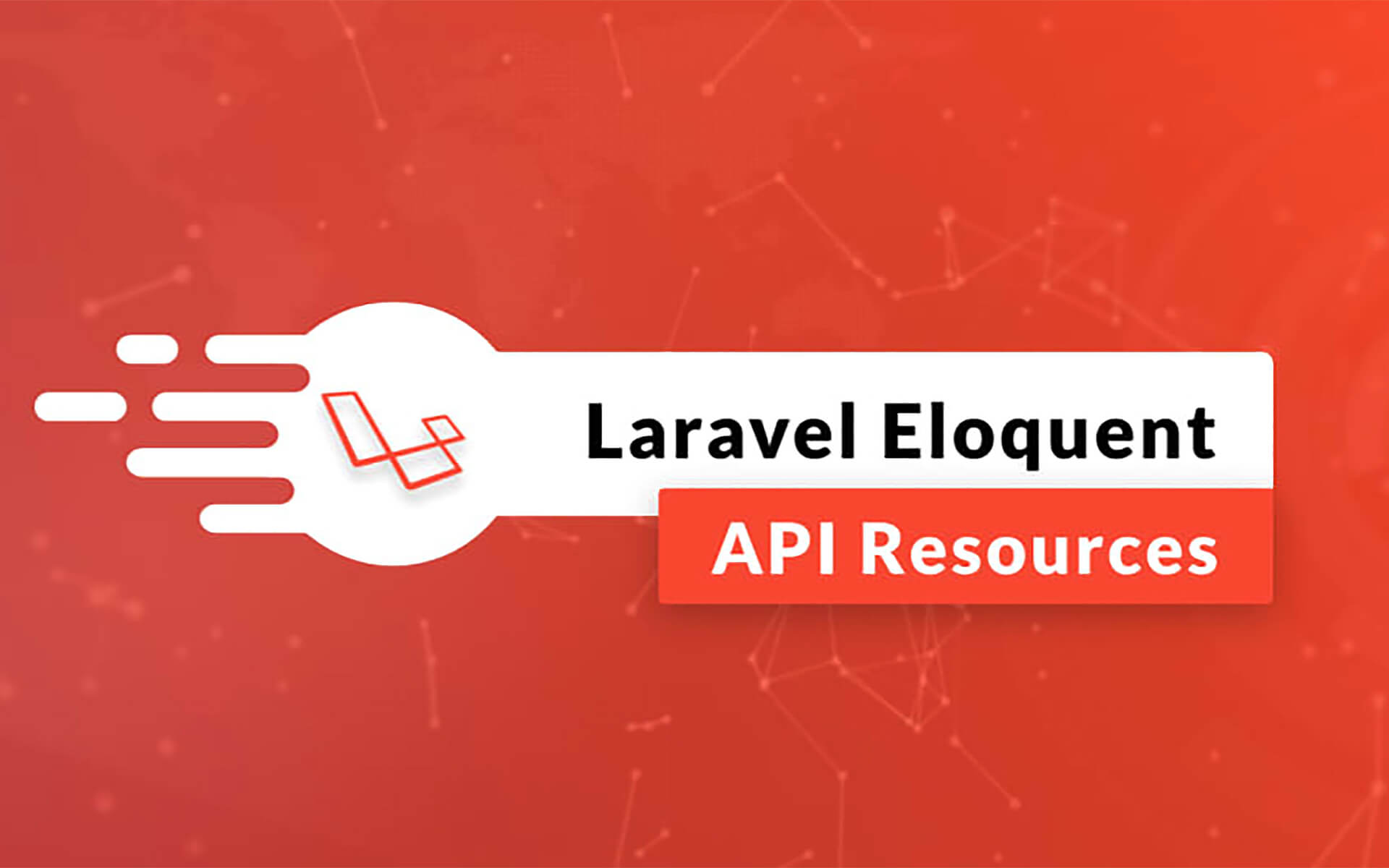
Following on from my previous blog Post (found here) we are going to be discussing two interesting parts of API Resources.
Using Resources to build up a data object
This is one of my favourite parts of using API Resources. Most people assume resources are only used for building up responses, but they are mistaken. I have used resources to build up JSON data objects for when interacting with external (or even internal) APIs.
You create the resource just as you normally would:
$fridge = Fridge::where(‘model’, $request->model)->where(‘black_friday_sale’, true)->first(); $fridge_resource = json_encode(FridgeResource::make($fridge));
And this should build up a brilliant JSON encoded Fridge Resource
. I feel doing this helps with a lot when it comes to managing your APIs. Having to deal with just one file makes life so much easier.
Don’t forget to put FridgeResource::withoutWrapping()
to get rid of the ‘data’ key wrapping the response!
Conditional Attributes
Conditional attributes are really interesting for me. If I have a model, let’s call it Fridge
, and I’m sending a response to more than one person (depending on their role) I would typically have some sort of nested folder structure like so:
Fridge > Manager > FridgeResource.php
Fridge > Customer > FridgeResource.php
It’s the same file, but with some differences. Perhaps for my Manager I want to show the amount of sales for that specific fridge, or when it’ll go on sale, or what the purchase price is/was, and you don’t want your customers seeing that sort of info!
So we can use Conditional Attributes in our resources. We can utilise the when
helper method that Laravel has so lovingly provided for us.
return [ // Regular fridge data ‘name’ => $this->name, ‘model-no’ => $this->model_no, ‘price’ => $this->price, ‘weight’ => $this->weight, ‘on-sale-black-friday’ => $this->black_friday_sale, ‘black-friday-discounted-price’ => $this->discount_price, // Only for managers ‘sales-today’ => $this->when(Auth::user()->isManager(), $this->getSales()), // Only for admins ‘self-destruct’ => $this->when( Auth::user()->isAdmin(), url(“self-destruct/{$this->id}”) ), ];
If the condition is true, then the fields are displayed in the response. If the user is not an admin, or a manager the `sales-today` and `self-destruct` keys will be completely removed from the response.
If you have multiple attributes you want to keep hidden you can surround them with the mergeWhen
method:
$this->mergeWhen(Auth::user()->isManager(), [ ‘sales-today’ => $this->getSales(), ‘next-sale-date’ => $this->next_sale_date, ])