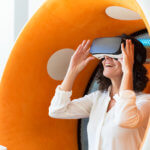
Virtual Reality: Game Changer in Education
June 13, 2023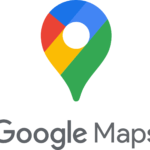
ASP.NET and Google Maps
June 26, 2023
Introduction:
Vue 3, the latest version of the popular JavaScript framework, introduces a powerful feature called the Composition API. This new API enhances code organization and reusability by providing a more flexible and expressive way to handle reactivity and component composition. In this blog post, we will explore the Vue 3 Composition API and learn how it can revolutionize your Vue development workflow.
What is the Composition API?
The Composition API is a feature in Vue.js 3 that offers a more flexible and scalable way to organize and reuse component logic compared to the traditional Options API. It encourages code reusability, better organization, and improved TypeScript support, making it easier to build and maintain complex applications.
Reactivity in Vue 3
Reactivity in Vue.js 3 enables dynamic updates in the user interface. Three main reactive primitives are available:
Refs: They hold reactive values. Example:
import { ref } from 'vue'; const count = ref(0); console.log(count.value); // 0 count.value++; // Triggers UI updates
Computed Properties: They derive values from reactive dependencies. Example:
import { ref, computed } from 'vue'; const firstName = ref('John'); const lastName = ref('Doe'); const fullName = computed(() => { return firstName.value + ' ' + lastName.value; }); console.log(fullName.value); // "John Doe" firstName.value = 'Jane'; // Updates fullName console.log(fullName.value); // "Jane Doe"
Watchers: They perform custom logic on changes. Example:
import { ref, watch } from 'vue'; const count = ref(0); watch(count, (newValue, oldValue) => { console.log('Count changed from', oldValue, 'to', newValue); }); count.value++; // Triggers the watcher callback
Component Composition with the Composition API
The Composition API in Vue.js 3 facilitates component composition by providing composition functions and lifecycle hooks. Composition functions allow for modular and reusable component logic, while lifecycle hooks offer control at different stages of a component’s lifecycle. Reactive component state is handled efficiently using reactive primitives like ref, reactive, and computed, enabling automatic UI updates when state changes. Overall, the Composition API simplifies the management of complex component logic in a more organized and flexible manner.
Advantages of the Composition API
The benefits of using the Composition API in Vue.js 3 include improved code organization, increased reusability through composition functions, better TypeScript support with enhanced type inference, enhanced readability and maintainability, and simplified testing. These advantages contribute to a more efficient development process, especially for building complex applications.
Migrating from Options API to Composition API
- Familiarize yourself with Vue 3 and the Composition API.
- Plan the migration strategy, choosing between gradual or complete migration.
- Identify components for migration and start with simpler ones.
- Extract reusable logic from Vue 2 components.
- Rewrite components using the Composition API, leveraging the extracted logic.
- Handle reactivity and data migration using reactive primitives.
- Test and refactor the migrated components, addressing any issues.
- Repeat the process for the remaining components.
Take a look at the vue documentation, use migration tools, and follow best practices to ensure a successful migration while maximizing the reuse of existing logic.
Limitations and Considerations
- Learning Curve: Transitioning from the Options API to the Composition API requires learning new concepts and syntax. Developers familiar with Vue 2 may need time to adjust to the composition function approach and understand the reactive primitives. It may take some time to become proficient in using the Composition API effectively.
- Tooling and Ecosystem Support: While Vue.js 3 has gained significant adoption, some existing Vue 2 ecosystem tools, libraries, and plugins may not have full support for the Composition API. This can pose challenges when integrating third-party tools or relying on existing Vue 2-specific libraries. However, the Vue.js community has been actively updating and creating new libraries and tools to support the Composition API.
- Compatibility with Existing Codebase: Migrating existing Vue 2 codebases to the Composition API may require significant changes to the component structure and logic. Some components may need to be rewritten entirely, and the process could be time-consuming, especially for large projects. It’s important to assess the impact on existing code and plan the migration strategy accordingly.
- Limited Resources and Examples: As the Composition API is relatively new compared to the Options API, there may be fewer resources, tutorials, and examples available specifically for the Composition API. Finding answers to specific questions or troubleshooting issues may require more effort initially, although the available resources are steadily growing.
- Developer Familiarity: The Composition API deviates from the traditional Vue.js development approach, and developers who are more experienced with the Options API may need to adjust their coding habits and patterns. This adjustment may take time and can potentially introduce inconsistencies if developers mix both approaches within the same codebase.
- Ecosystem Fragmentation: The introduction of the Composition API can lead to a fragmented ecosystem, with some developers using the Composition API and others continuing to use the Options API. This can make it challenging to maintain consistency and share code across projects or teams.
Conclusion:
The Vue 3 Composition API empowers developers to create maintainable and reusable Vue applications. It enables reactivity and component composition, leading to better code structure and organization. Embrace the Composition API to unlock a more elegant and efficient development experience in your Vue projects.
I hope some of you have found this blog helpful.