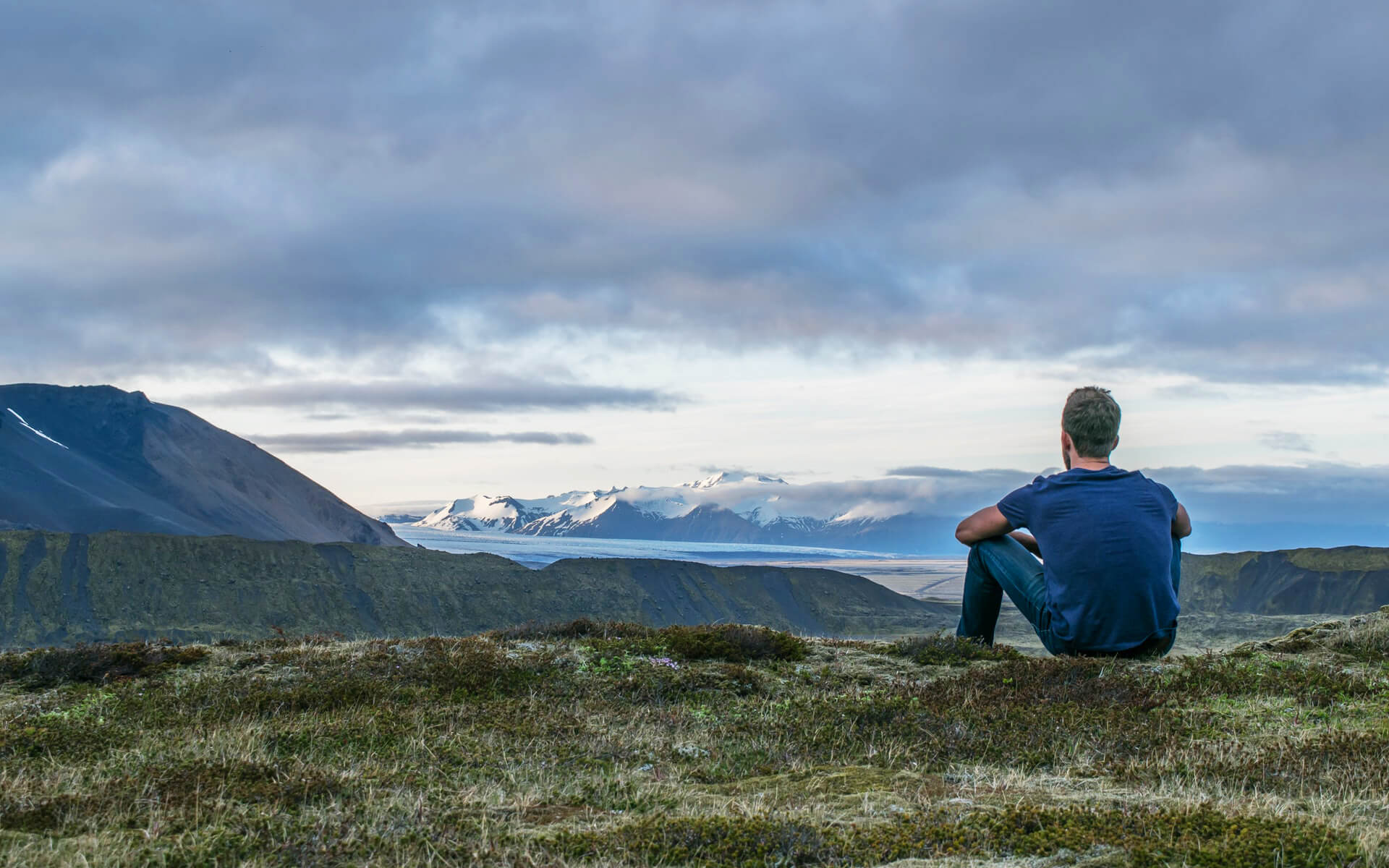
Imposter Syndrome
June 20, 2022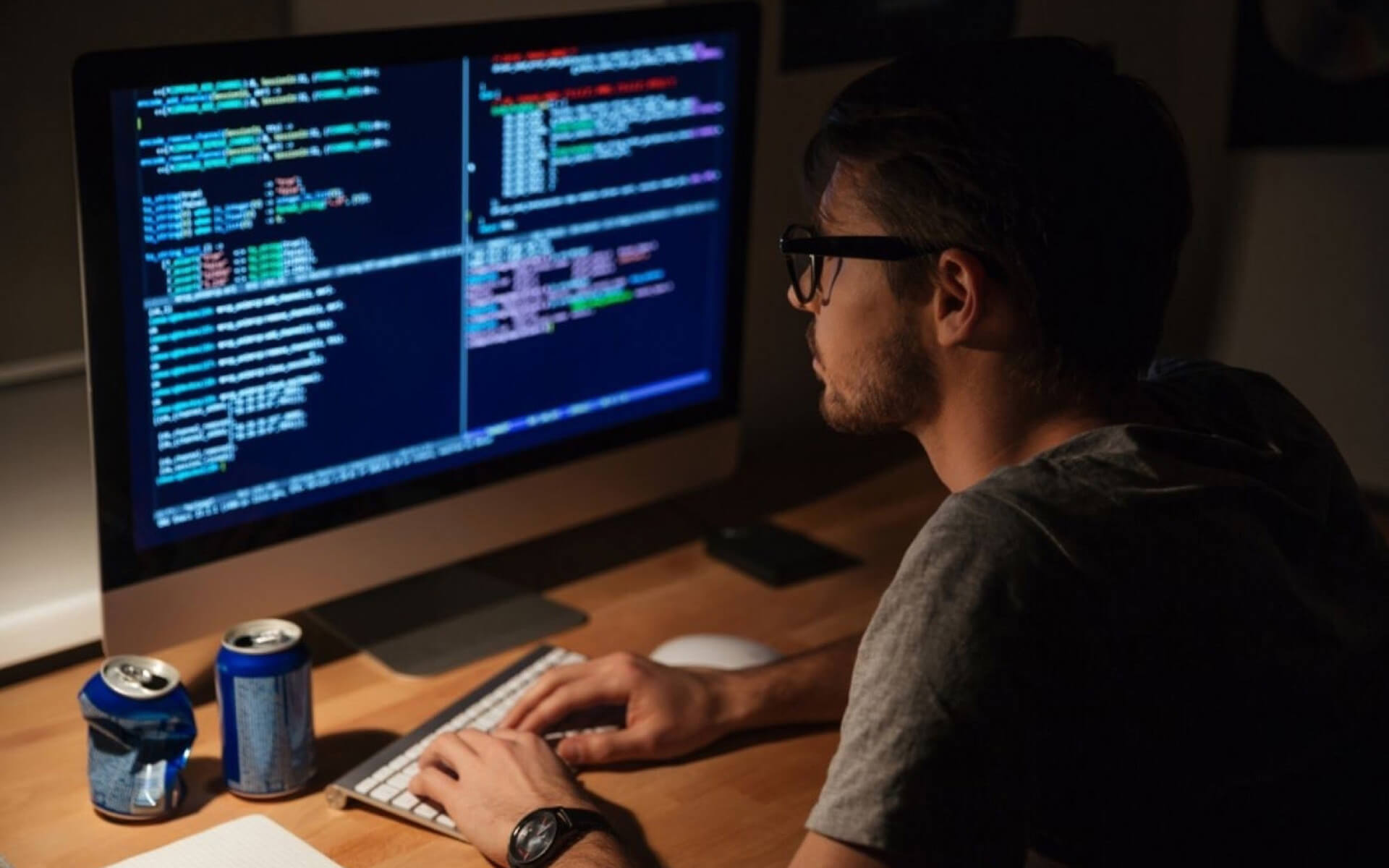
Exposure To New And Unfamiliar Things
July 8, 2022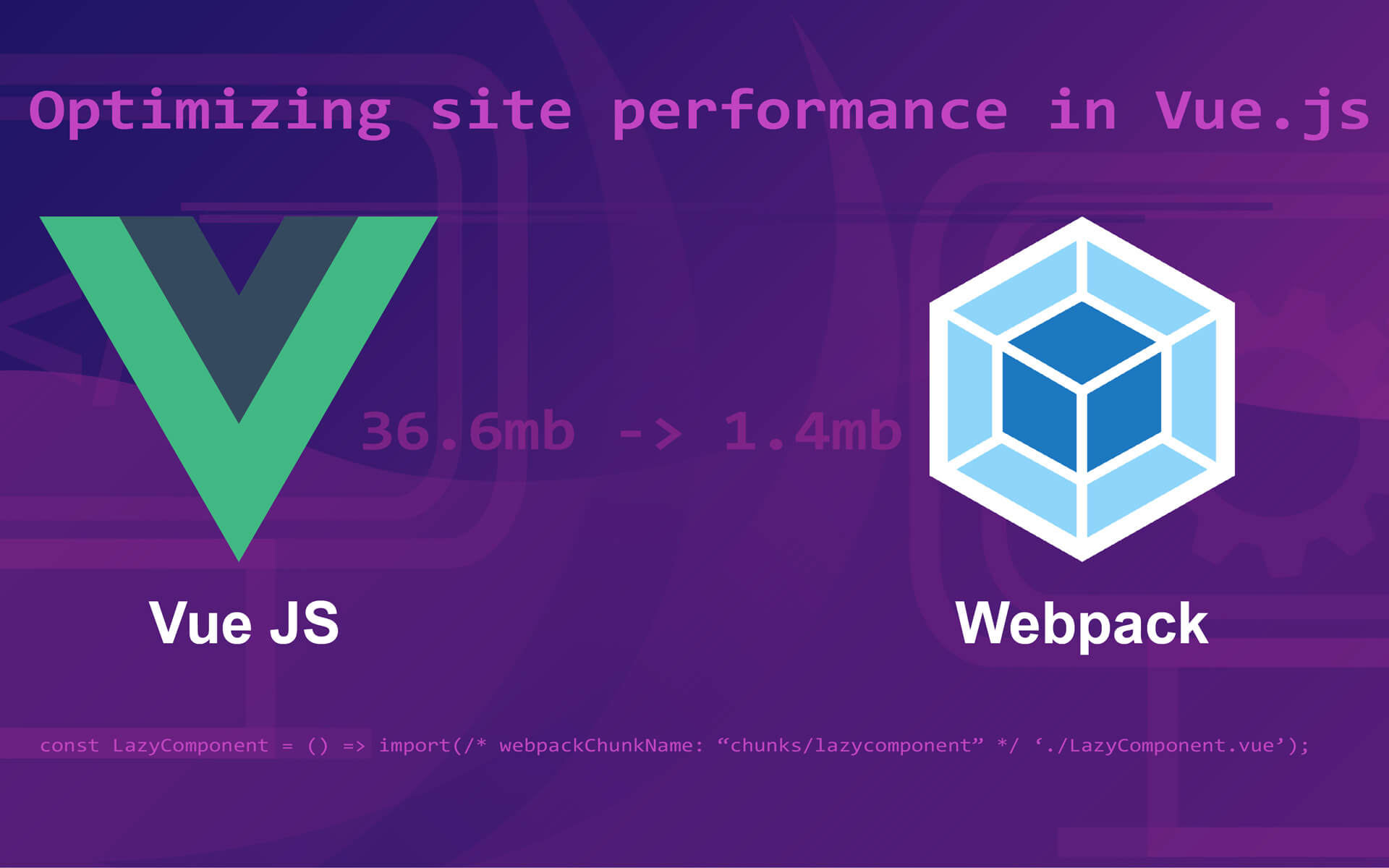
An issue every developer will face at least once in their career is making your app or website load and run faster. This however is not always a simple task, and most people have no idea where to even start looking.
I have listed a couple solutions below with little titbits of information just to help you get started on your venture down the deep rabbit hole of site optimisation with an emphasis on Vue.js.
Module Bundlers
A common practice for reducing the size of any javascript-based application is using a module bundler. There are quite a few that exist but by the most popular and commonly used is Webpack.
Webpack consolidates all your code and any external libraries into a single javascript file that can be referenced at the header of your application.
While this is great since it means everything you need can be tucked neatly in a single place, it also affects the initial load time of your application significantly since as the project grows in scope, the size of the bundled file grows exponentially bigger as well which can become problematic with slow internet speeds.
Here are a couple solutions to help mitigate this problem:
Lazy-Loading
The term lazy-loading in software engineering refers to the pattern of deferring the initialisation of an object or a part of an application until it is needed.
Usually when we’re bundling our application, it results in one large Javascript file. However by lazy-loading our components, the code is split into the main file and smaller files called “chunks”, that are referenced by our bundler so it only needs to be loaded when the component is needed instead of at the get-go.
The syntax for lazy loading components in VueJS is as follows:
const LazyComponent = () => import('./LazyComponent.vue');
Code Splitting
One thing you may notice however is that this results in a file being generated with a random name and for every component that is lazy-loaded a new random file gets generated. This however feels like we’re just reverting back to our application before introducing webpack in the first place.
But we can get around this issue by assigning our imports a chunkName. This bundles all the code with the same chunkName into the same chunk, this also has the added benefit of ensuring that all related components get loaded in at the same time.
We achieve this by using “magic comments” to assign the chunkName on the code that is being imported.
Example:
const LazyComponent = () => import(/* webpackChunkName: "chunks/lazycomponent" */ './LazyComponent.vue');
Here the chunk will be generated with the name “lazycomponent” within a folder called “chunks”.
Prefixing the chunkName with the folder is simply to allow for better use when adding the file to your .gitignore file and is not necessary.
If you want more in-depth explanation about how this works I highly recommend checking out these resources: