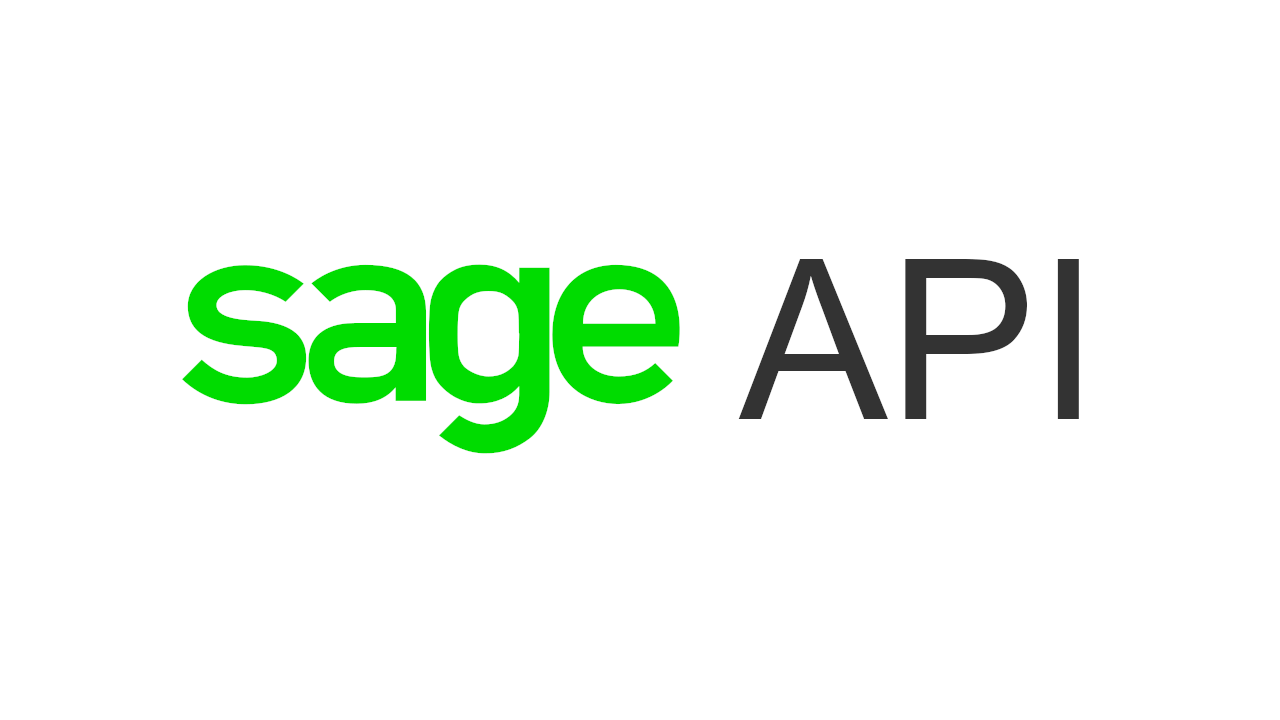
Getting Your Sage One Company ID For API Integration
September 6, 2019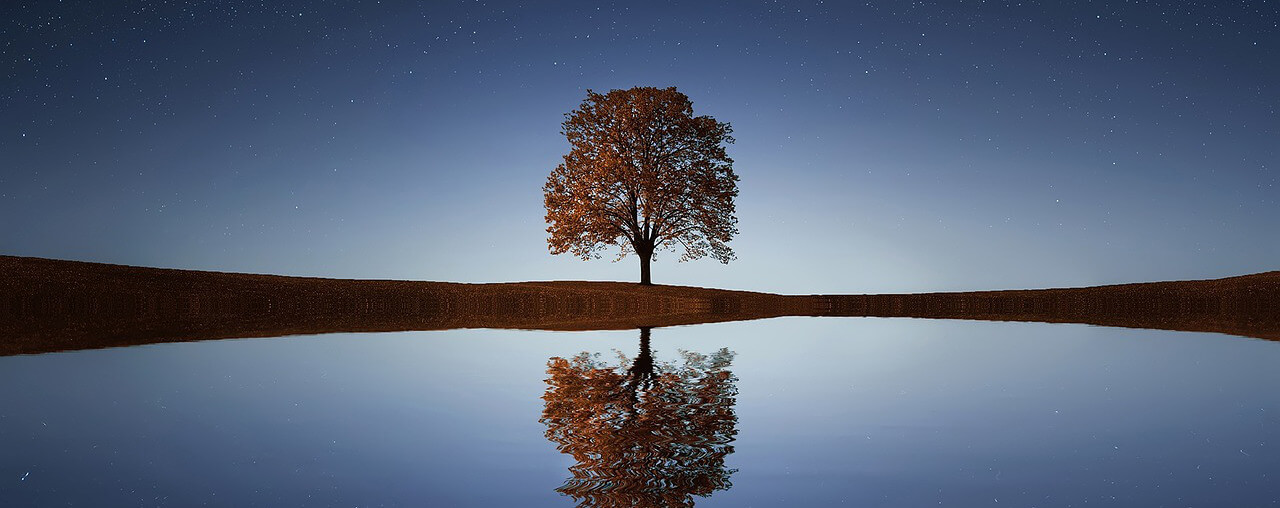
System Environment Variables On Windows
September 18, 2019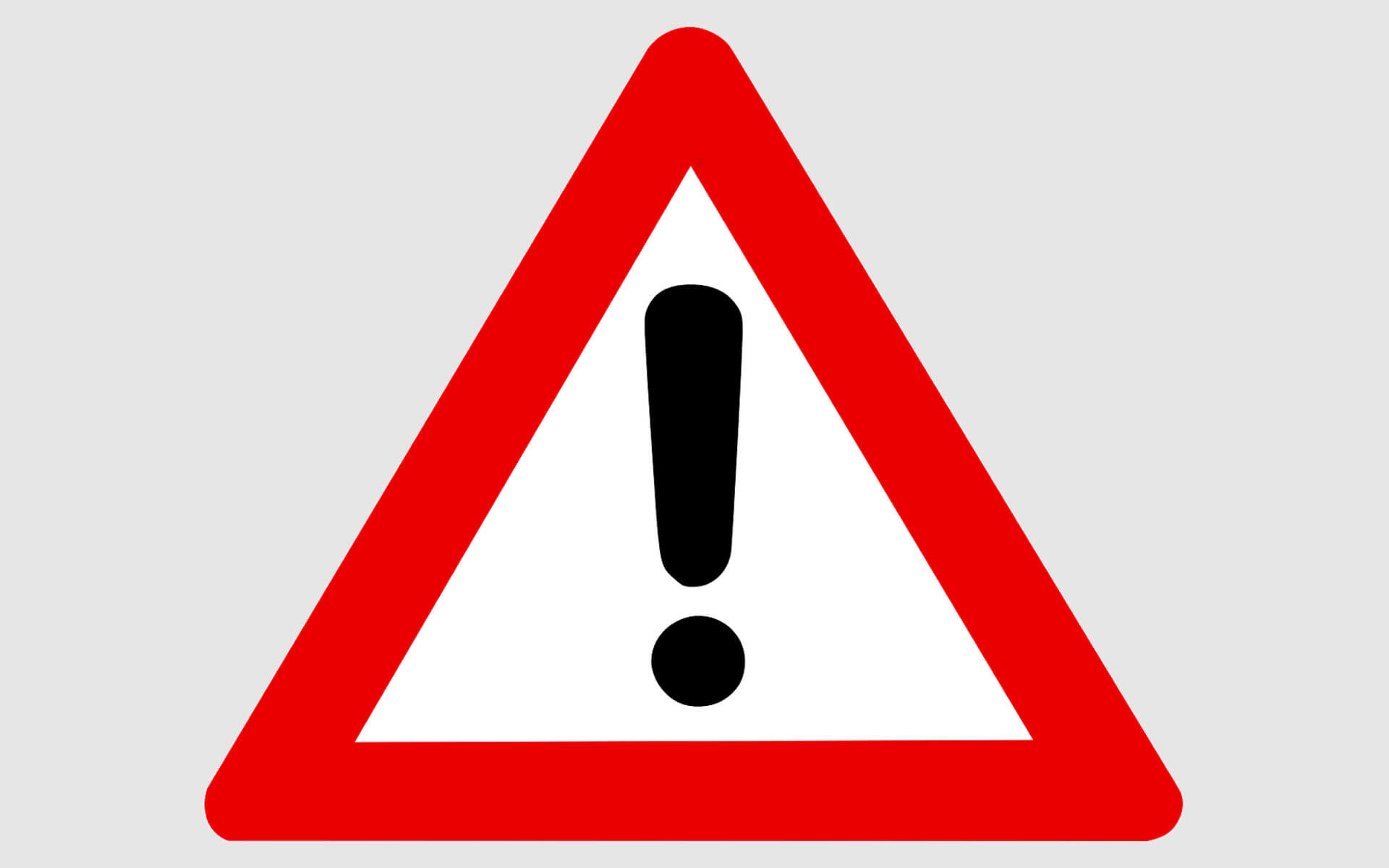
Inspired by the Scala Try class, I thought there must be an easy way to make a Java function that accepts a lambda as an argument and returns the result of calling the lambda or null if the lambda throws an exception. Here it is:
public class ExceptionUtil { public interface MayThrow{ public R method() throws Exception; } public static T tryBlock(MayThrow throwing) { try{ T obj = throwing.method(); return obj; } catch(Exception e) { System.out.println(e.getLocalizedMessage()); return null; } } }
This is an example of how you use it:
Double number = ExceptionUtil.tryBlock(() -> Double.valueOf(numberAsString))
If numberAsString doesn't parse into a double an exception is thrown and the exception is handled in the tryBlock method and null would be returned.
The full class with Java Optional return
If you would like it to return a Java Optional instead, here is the full class with tryBlockOptionalMethod:
import java.util.Optional; public class ExceptionUtil { public interface MayThrow{ public R method() throws Exception; } public static T tryBlock(MayThrow throwing) { try{ T obj = throwing.method(); return obj; } catch(Exception e) { System.out.println(e.getLocalizedMessage()); return null; } } public static Optional tryBlockOptional(MayThrow throwing) { try{ return Optional.ofNullable(throwing.method()); } catch(Exception e) { return Optional.empty(); } } }