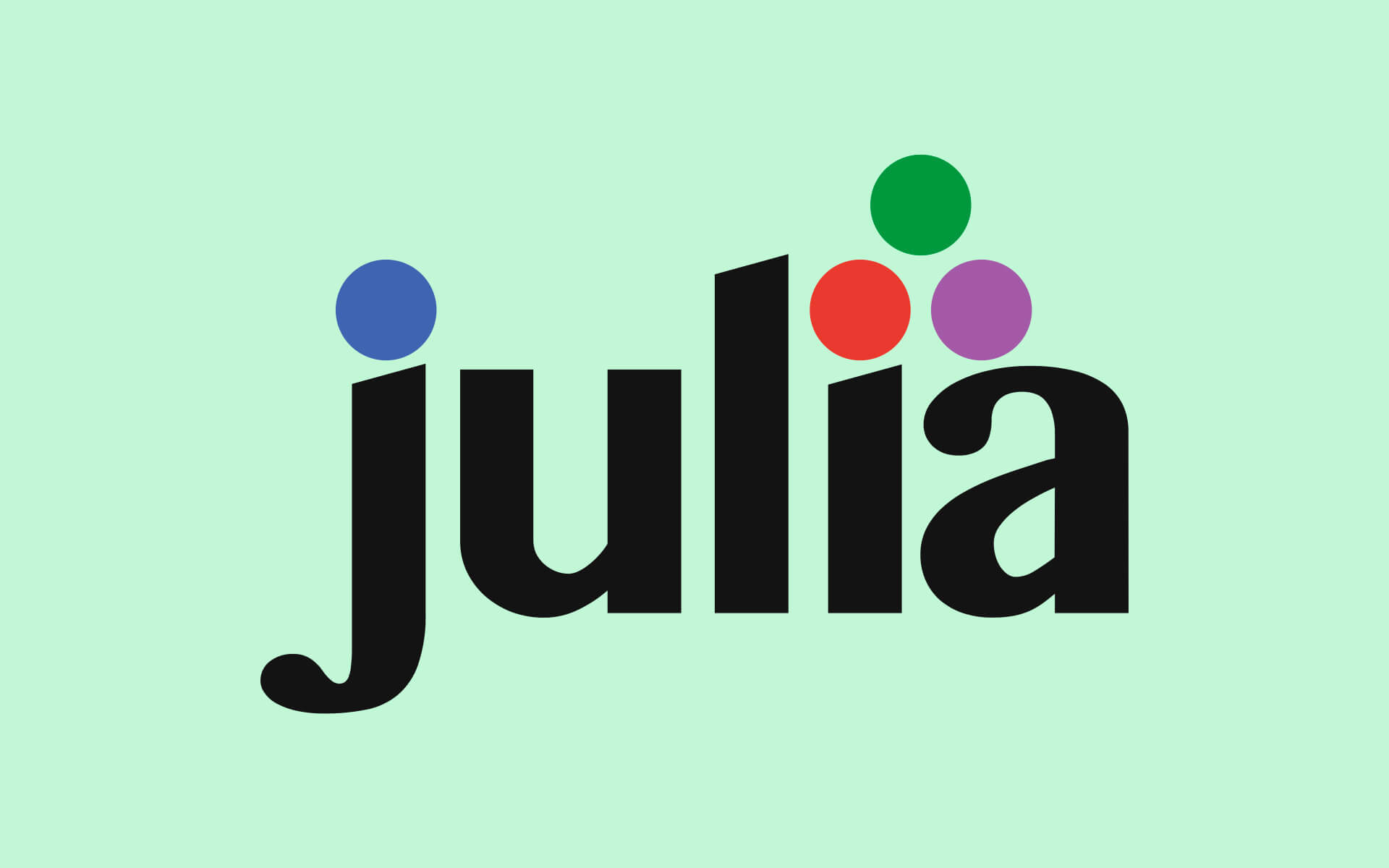
Julia Programming Language
November 7, 2022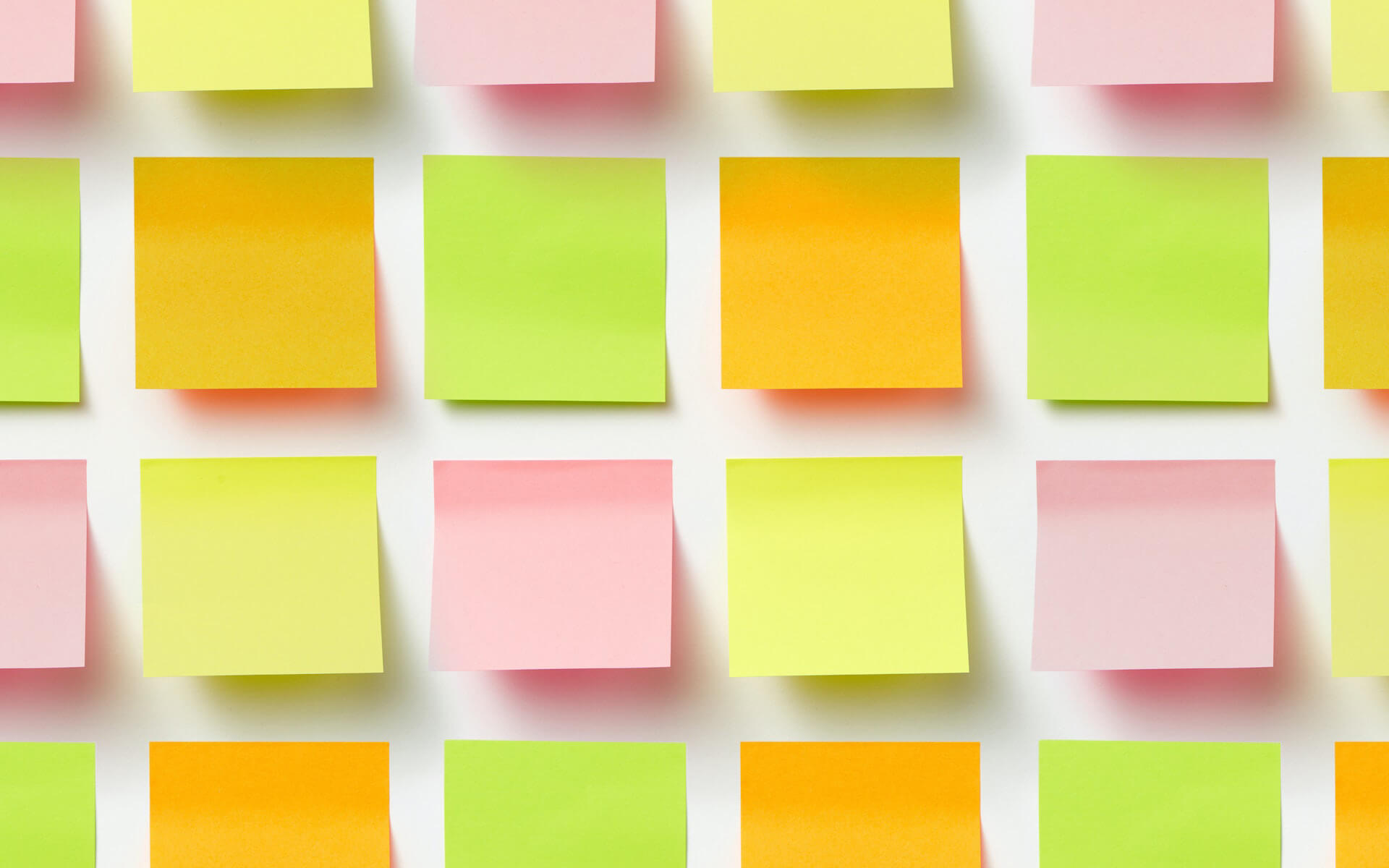
How To Create An Angular Form Array
December 5, 2022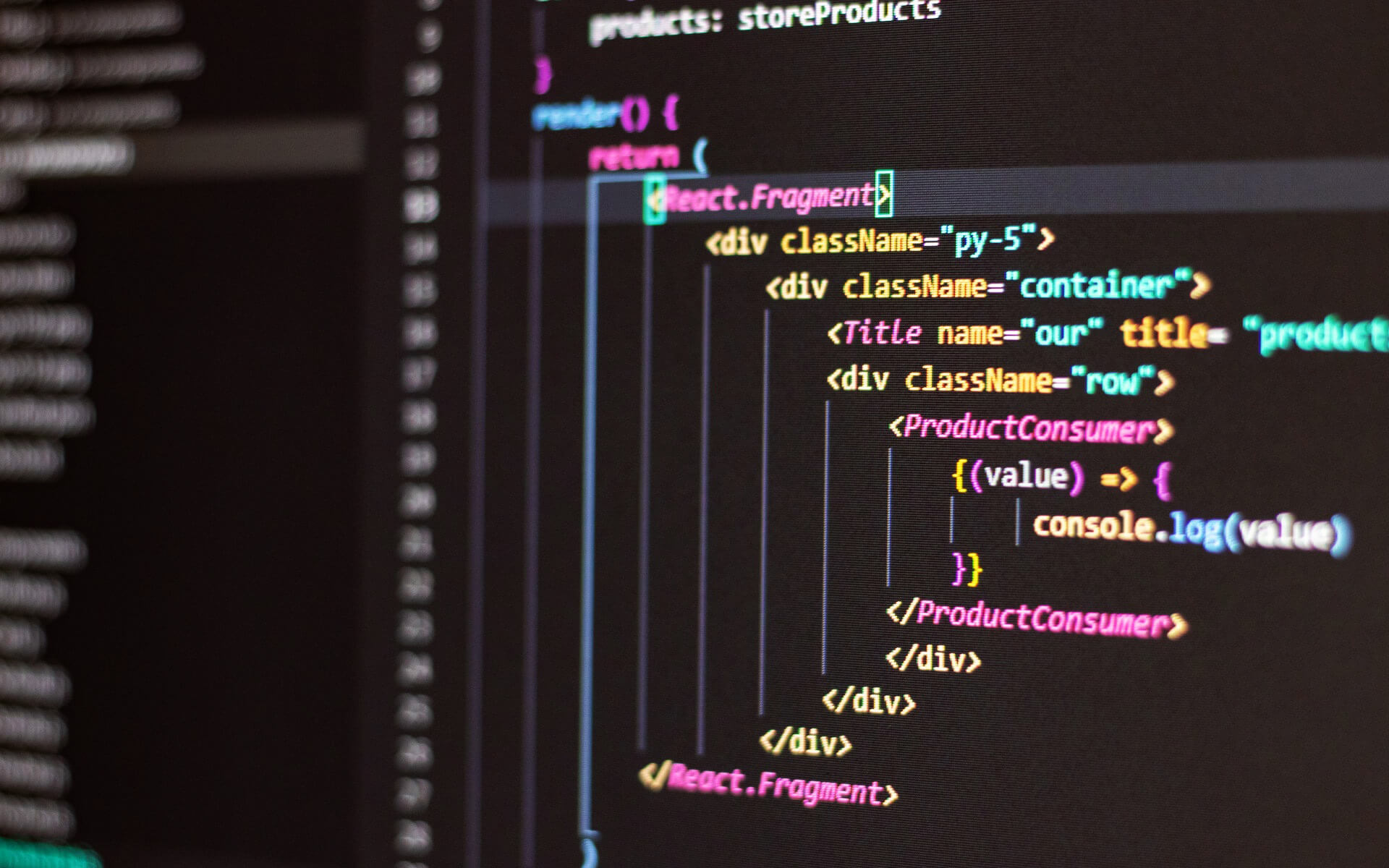
Introduction
Once you have started using and creating your own custom snippets in Visual Studio code, you may start encountering use cases where you want to use more advanced features or wish that certain behaviours could be altered.
Customising Snippet Behaviours
Tab completion provides an alternative method of invoking snippets to the standard Intellisense. when enabled by the editor.tabCompletion, press Tab to insert a snippet with the prefix you typed. This will need to be enabled only for snippets or in general, as it is off by default.
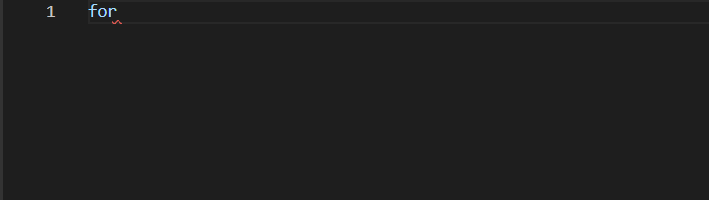
Further, you can customize where snippets appear in the Intellisense menu; this is changed by the editor.snippetSuggestions setting. By default, it is mixed with other suggestions, but you can arrange for them to be grouped at the top or bottom, or even completely removed from the suggestions.
An example of the default snippet suggestion

An example of the snippet suggestion set to top

An example of the snippet suggestion set to bottom
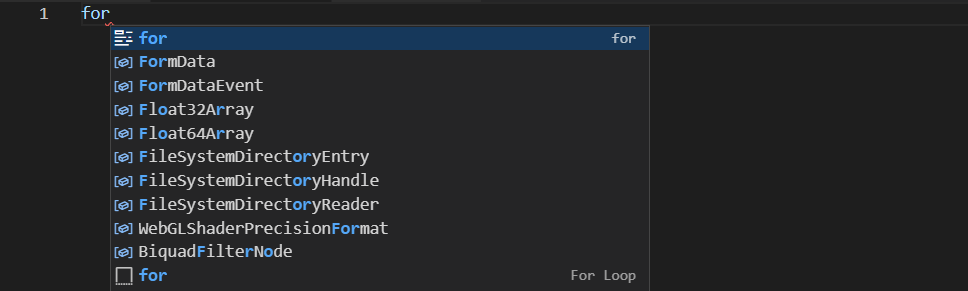
An example of when the snippet suggestion set to disabled
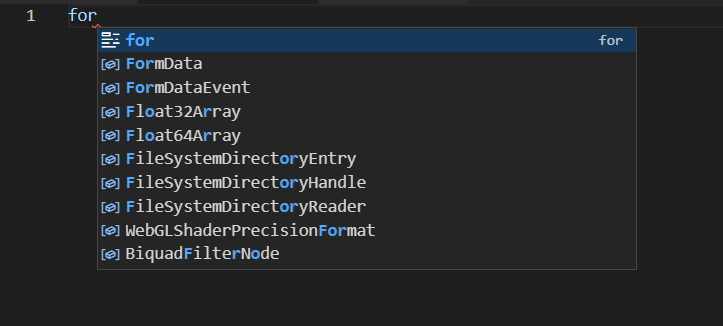
Snippet Variables
VS Code snippets allow you to use predefined variables to increase the flexibility of the snippets. Like tab stops, it is prefixed with a dollar sign: $TM_LINE_NUMBER
, and you can use the curly brackets with a colon to define a default if the variable is empty: ${CLIPBOARD:hello world}
Selected Text Variable
The variable that I use the most is the selected text variable. $TM_SELECTED_TEXT
It allows you to use the text you have highlighted in the snippet. This has many uses, for example, putting your highlighted text into a try catch.
"Try Catch": {
"prefix": "try_catch",
"body": [
"try {",
"\t${TM_SELECTED_TEXT:${1:$LINE_COMMENT code ...}}",
"} catch (${2:\\Throwable} ${3:\\$th}) {",
"\t${4:$LINE_COMMENT throw \\$th;}",
"}",
"$0"
],
}
To make the most effective use of the selected text snippet variable, you have two options.
The first is to assign the snippets to a keyboard shortcut. The easiest way to do this is by going to the keybindings. json via File > Preferences > Keyboard Shortcuts and then clicking on the icon on the top right.
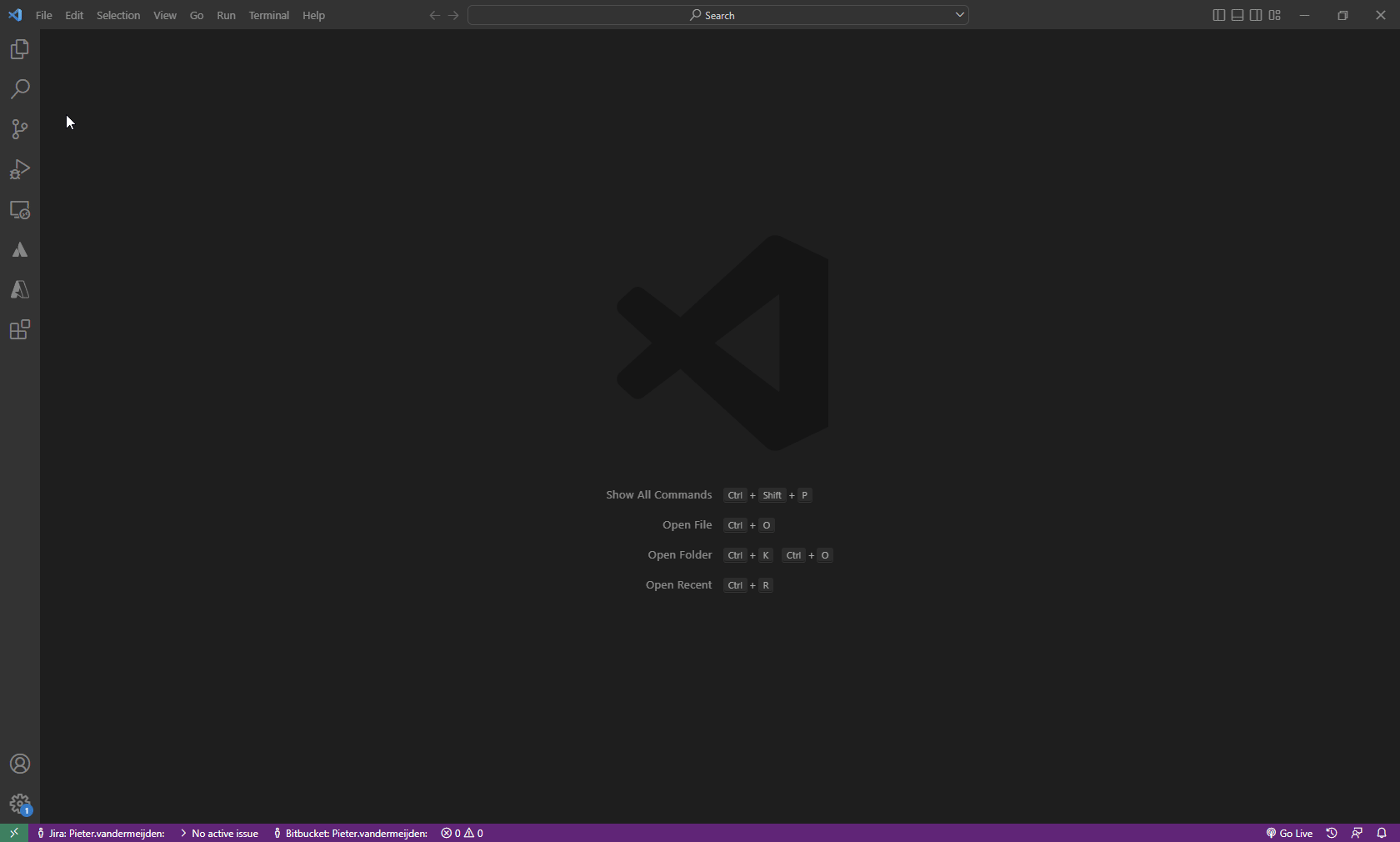
Then you can create a shortcut using json, for example, that will assign the try-catch snippet to ctrl+alt+t.
{
"key": "ctrl+alt+t",
"command": "editor.action.insertSnippet",
"args": { "name": "try_catch" }
}
The second way of using the selected text variable snippets was introduced in the 1.70 (July 2022) update’s code action rework. To use it, create a snippet with the selected text variable and then press ctrl +. to open a context menu where you can select that snippet.
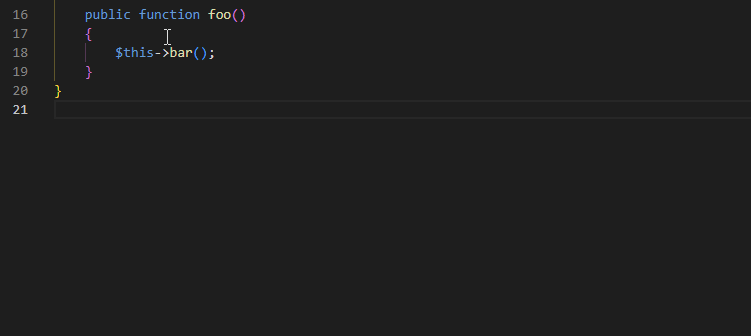
The Clipboard Variable
It is a common joke that programming is little more than copy-pasting from stack overflow; regardless of the programmer's experience, one will find that they are copy-pasting from API documentation, etc. This can be combined with VS Code’s $CLIPBOARD
variable to allow you to format your copied text with snippets. A simple example would be to paste it with double quotes.
"String paste": {
"prefix": "str",
"body": [
"\"${1:$CLIPBOARD}\"$0"
],
"description": "allows copied text to be surrounded using double quotes"
}
Comment Variables
If you use snippets to create comments, you will find it annoying to have to create a snippet for every language that you use. Fortunately, there are variables that will insert the correct comment syntax for the current language. This allows you to simply create the snippet globally and use it for all your languages.
For an in-line comment, you can use the $LINE_COMMENT
variable; a common use case would be a TODO comment.
"todo": {
"prefix": "todo",
"body": ["$LINE_COMMENT TODO ${1:remove}", "$0"],
"description": "add a todo comment"
}
Also, there are variables for block comments. $BLOCK_COMMENT_START
opens the block comment, and all the following lines will be formatted correctly to be in the block comment, for example, by prefixing them with asterisks in PHP. The block comment can then be closed with the $BLOCK_COMMENT_END
variable.
"Insert Doc block": {
"prefix": "doc_block",
"body": [
"$BLOCK_COMMENT_START",
"@author Pieter van der Meijden",
"$1",
"$BLOCK_COMMENT_END"
]
},
Date Time Variables
Snippet variables can also be used to output the current date and time in the format that you would like. First, you can get the full year with $CURRENT_YEAR
or just the last two digits with $CURRENT_YEAR_SHORT
. For the month, you can get it as a number with $CURRENT_MONTH
, the name with $CURRENT_MONTH_NAME
, or the shortened name with $CURRENT_MONTH_NAME_SHORT
. The day of the month is simply the $CURRENT_DATE
variable, while the day of the week has a full name with $CURRENT_DAY_NAME
and a short variant with $CURRENT_DAY_NAME_SHORT
. For the time, it can be outputted in 24-hour format using the $CURRENT_HOUR
, $CURRENT_MINUTE
, and $CURRENT_SECOND
variables. If needed, there is also Unix time $CURRENT_SECONDS_UNIX
. An use case for the date time variables would be adding it to a doc block.
"Insert Doc block": {
"prefix": "doc_block",
"body": [
"$BLOCK_COMMENT_START",
"@author Pieter van der Meijden",
"@date $CURRENT_DATE-$CURRENT_MONTH_NAME_SHORT-$CURRENT_YEAR $CURRENT_HOUR:$CURRENT_MINUTE:$CURRENT_SECOND",
"$1",
"$BLOCK_COMMENT_END"
]
}
File Variables
Sometimes you want to use the file name that you are working with for doc blocks, and VS Code allows you to extract this and more in your snippets with the following variables: You can use $TM_FILENAME
for the file name with the extension and $TM_FILENAME_BASE
for the name without. If you want the path for the current file, use $TM_DIRECTORY
, or if you want the file name with the extension appended, use $TM_FILEPATH
. Additionally, you can get the current VS Code workspace using $WORKSPACE_NAME
.
Line Variables
Another set of useful variables for extracting information about the line that you are currently on you can get the number of the line for either a zero or nonzero index with the $TM_LINE_INDEX
and $TM_LINE_NUMBER
variables, respectively. Also, you can get the contents of the line using the $TM_CURRENT_LINE
variable, or for simpler snippets, you can just get the word that is underneath the cursor using the $TM_CURRENT_WORD
variable.