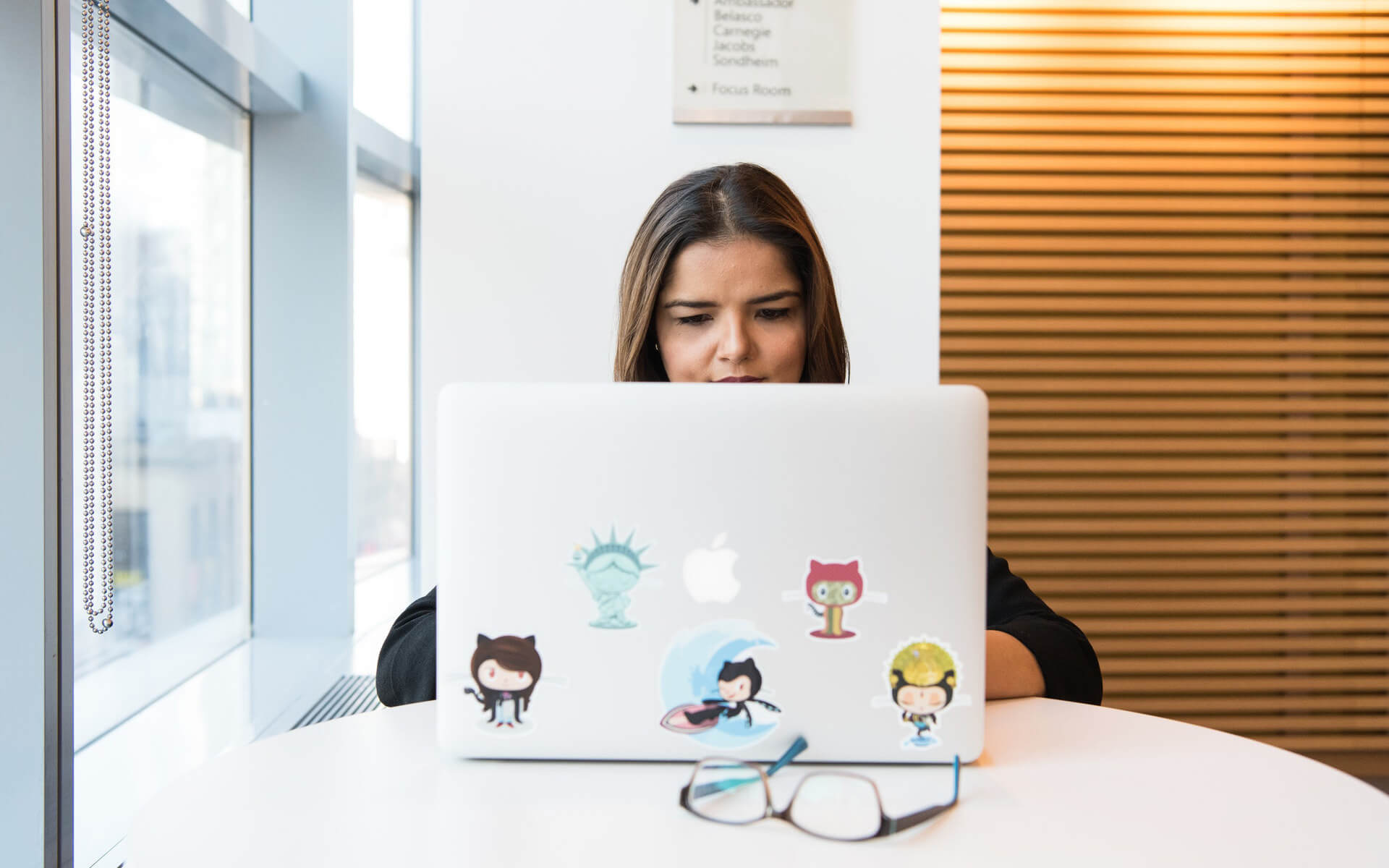
How To Setup Git-ftp For Windows
June 29, 2021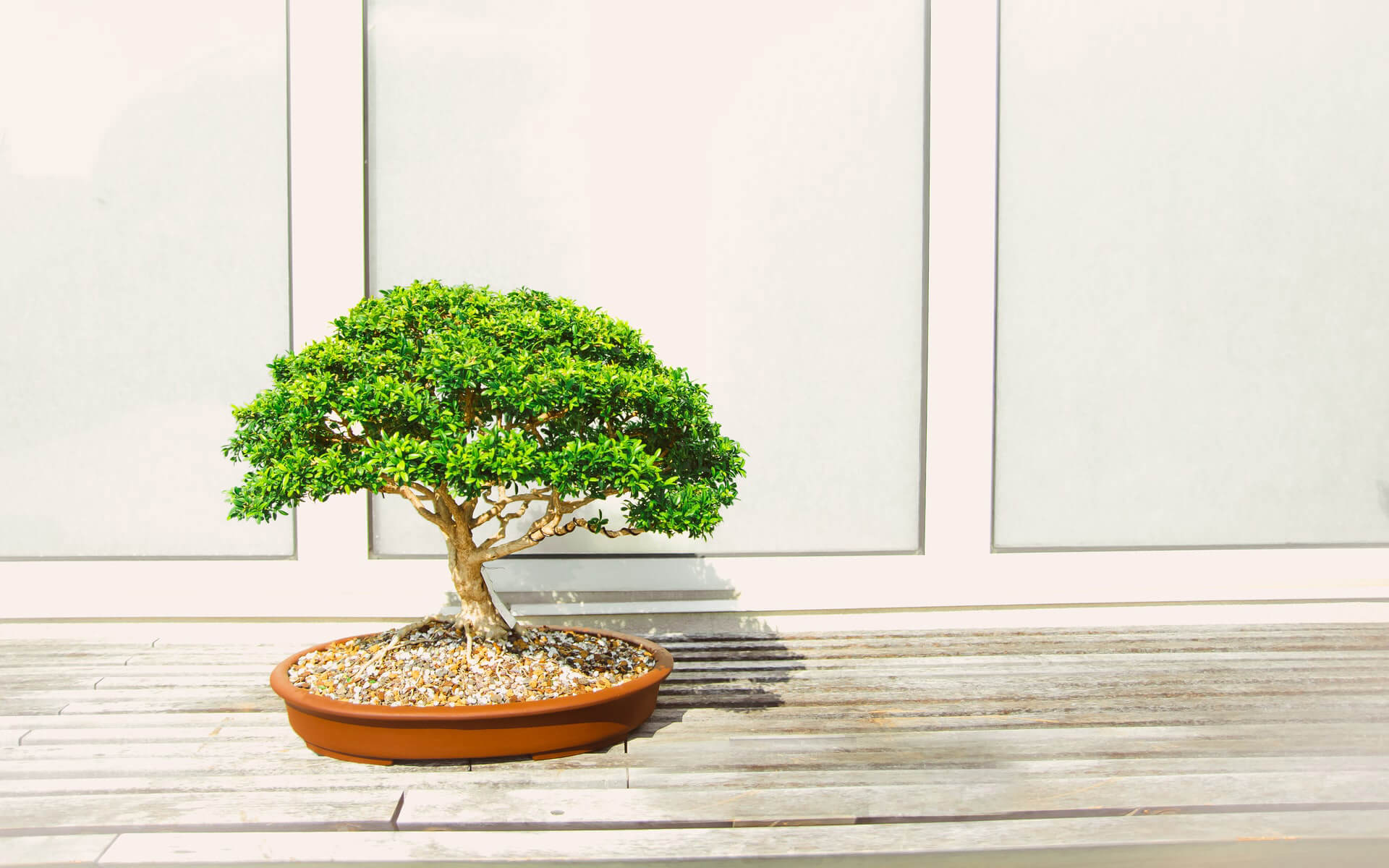
A Developers Bonsai
July 12, 2021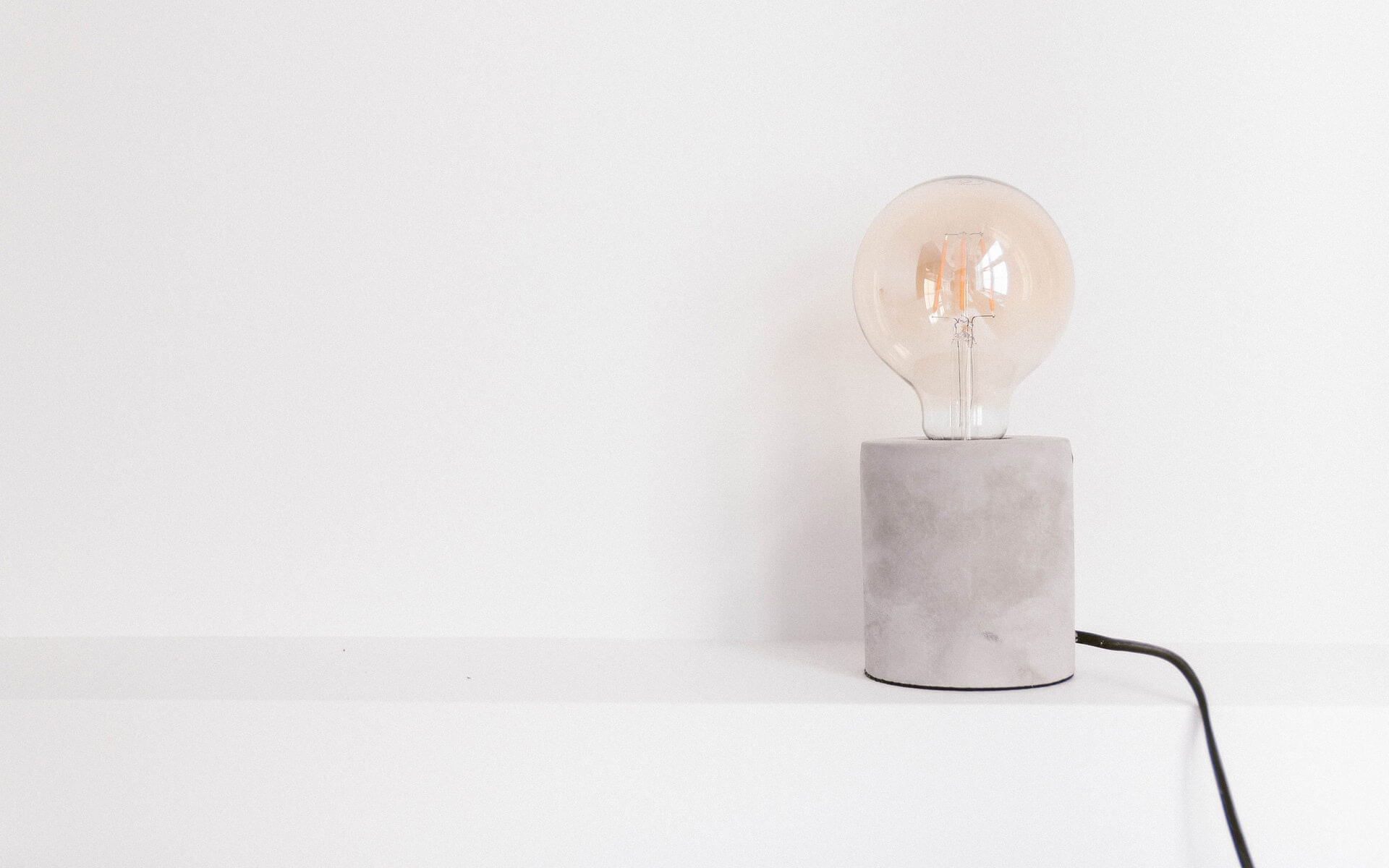
Laravel Livewire is a library that makes it easy to create applications in a fast, efficient manner. With it, the data displayed on the front end becomes reactive, and you are able to affect it and manipulate it without having to leave your page. It does this by making use of Modals which interact with your Backend, without you having to POST data to it.
The Flow
As stated above, Livewire makes use of Modals. In the below example, we make use of the dialog-modal
to create a Popup that will allow the User to add a Cat Fact. The Dashboard, where we load our Cat Facts into a table, is also a paginated list that is rendered by Livewire.
The Code
After installing Livewire using composer (using Jetstream or composer require calebporzio/livewire
), we’ll need to make our Livewire components.
php artisan make:livewire CatFacts
will create the Livewire components we need. We’ll find them at app/Http/Livewire/CatFacts.php
and resources/views/livewire/catfacts.blade.php
. By default, the CatFacts.php
Livewire component comes with many functions, including render
, store
, edit
, update
and destroy
. For this example, we’ll only be making use of the render
, create
and store
functions.
The render
function renders the actual Dashboard page. We pass it a paginated list of CatFacts.
The create
function controls the Popup Modal.
The store
function takes care of the creation of the CatFact.
Please see our final components below.
app/Http/Livewire/CatFacts.php:
namespace App\Http\Livewire;
use Livewire\Component;
use Livewire\WithPagination;
use App\Models\CatFact;
use GuzzleHttp\Client;
use Auth;
class CatFactsCrud extends Component {
use WithPagination;
public $modalOpen = false;
public function render()
{
return view('livewire.catfacts', [ 'catFacts' => CatFact::paginate(5) ]);
}
public function create()
{
$this->openModal();
}
public function openModal()
{
$this->modalOpen = true;
}
public function closeModal()
{
$this->modalOpen = false;
}
public function store()
{
$this->validate([
'fact_text' => 'required',
]);
$catFact = Catfact::where('fact_text', $this->fact_text)->first();
if (!$catFact) {
$catFact = new CatFact();
$catFact->fact_id = null;
$catFact->user_id = Auth::user()->id;
$catFact->fact_text = $this->fact_text;
$catFact->save();
session()->flash('message', 'Cat Fact successfully created.');
} else {
session()->flash('message', 'Cat Fact could not be created. Fact already exists.');
}
$this->closeModal();
}
}
resources/views/livewire/catfacts.blade.php:
<div class="py-12">
<div wire:loading class="fixed top-0 left-0 right-0 bottom-0 w-full h-screen z-50 overflow-hidden bg-gray-700 opacity-75 flex flex-col items-center justify-center">
<div class="loader ease-linear rounded-full border-4 border-t-4 border-gray-200 h-12 w-12 mb-4"></div>
<h2 class="text-center text-white text-xl font-semibold">Loading...</h2>
</div>
<div class="max-w-7xl mx-auto sm:px-6 lg:px-8">
<div class="bg-white overflow-hidden shadow-xl sm:rounded-lg px-4 py-4">
@if (session()->has('message'))
<div class="bg-blue-100 border-t border-b border-blue-500 text-blue-700 px-4 py-3" role="alert">
<div class="flex">
<p class="text-sm">{{ session('message') }}</p>
</div>
</div>
@endif
<div class="grid grid-cols-3 gap-4">
<div>
<button wire:click="create()" class="bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded my-3 float-right">
Create New Cat Fact
</button>
</div>
</div>
@if($modalOpen)
@include('livewire.create')
@endif
<table class="table-fixed w-full">
<thead>
<tr class="bg-gray-100">
<th class="px-4 py-2 w-20">ID</th>
<th class="px-4 py-2">Text</th>
<th class="px-4 py-2">User</th>
<th class="px-4 py-2">Updated At</th>
</tr>
</thead>
<tbody>
@foreach($catFacts as $catFact)
<tr>
<td class="border px-4 py-2">{{ $catFact->id }}</td>
<td class="border px-4 py-2">{{ $catFact->fact_text }}</td>
<td class="border px-4 py-2">
@if(isset($catFact->user))
{{ $catFact->user->name ?: '-' }}
@else
-
@endif
</td>
<td class="border px-4 py-2">{{ $catFact->updated_at }}</td>
</tr>
@endforeach
</tbody>
</table>
</div>
{{ $catFacts->links() }}
</div>
</div>
resources/views/livewire/create.blade.php:
<div class="fixed z-10 inset-0 overflow-y-auto ease-out duration-400">
<div class="flex items-end justify-center min-h-screen pt-4 px-4 pb-20 text-center sm:block sm:p-0">
<div class="fixed inset-0 transition-opacity">
<div class="absolute inset-0 bg-gray-500 opacity-75"></div>
</div> <!-- This element is to trick the browser into centering the modal contents. -->
<span class="hidden sm:inline-block sm:align-middle sm:h-screen"></span>
​<div class="inline-block align-bottom bg-white rounded-lg text-left overflow-hidden shadow-xl transform transition-all sm:my-8 sm:align-middle sm:max-w-lg sm:w-full" role="dialog" aria-modal="true" aria-labelledby="modal-headline">
<form>
<div class="bg-white px-4 pt-5 pb-4 sm:p-6 sm:pb-4">
<div class="">
<div class="mb-4">
<label for="exampleFormControlInput1" class="block text-gray-700 text-sm font-bold mb-2">Fact Text:</label>
<input type="text" class="shadow appearance-none border rounded w-full py-2 px-3 text-gray-700 leading-tight focus:outline-none focus:shadow-outline" id="exampleFormControlInput1" placeholder="Enter Fact Text" wire:model="fact_text">
@error('fact_text') <span class="text-red-500">{{ $message }}</span>@enderror
</div>
</div>
</div>
<div class="bg-gray-50 px-4 py-3 sm:px-6 sm:flex sm:flex-row-reverse">
<span class="flex w-full rounded-md shadow-sm sm:ml-3 sm:w-auto">
<button wire:click.prevent="store()" type="button" class="inline-flex justify-center w-full rounded-md border border-transparent px-4 py-2 bg-green-600 text-base leading-6 font-medium text-white shadow-sm hover:bg-green-500 focus:outline-none focus:border-green-700 focus:shadow-outline-green transition ease-in-out duration-150 sm:text-sm sm:leading-5"> Save </button>
</span>
<span class="mt-3 flex w-full rounded-md shadow-sm sm:mt-0 sm:w-auto">
<button wire:click="closeModal()" type="button" class="inline-flex justify-center w-full rounded-md border border-gray-300 px-4 py-2 bg-white text-base leading-6 font-medium text-gray-700 shadow-sm hover:text-gray-500 focus:outline-none focus:border-blue-300 focus:shadow-outline-blue transition ease-in-out duration-150 sm:text-sm sm:leading-5"> Cancel </button>
</span>
</form>
</div>
</div>
</div>
</div>
Conclusion
The final result should look like the below.