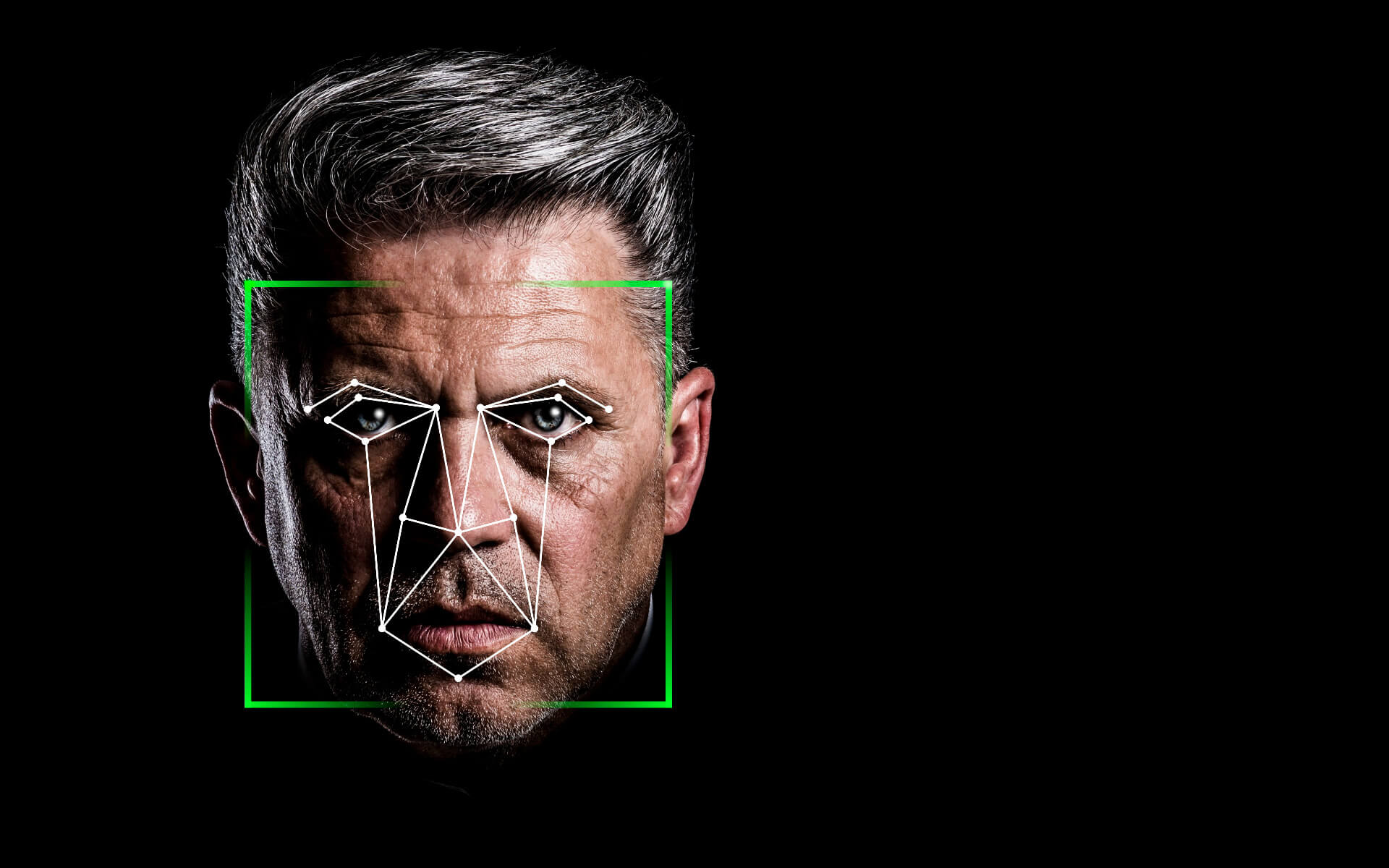
Computer Vision
October 18, 2021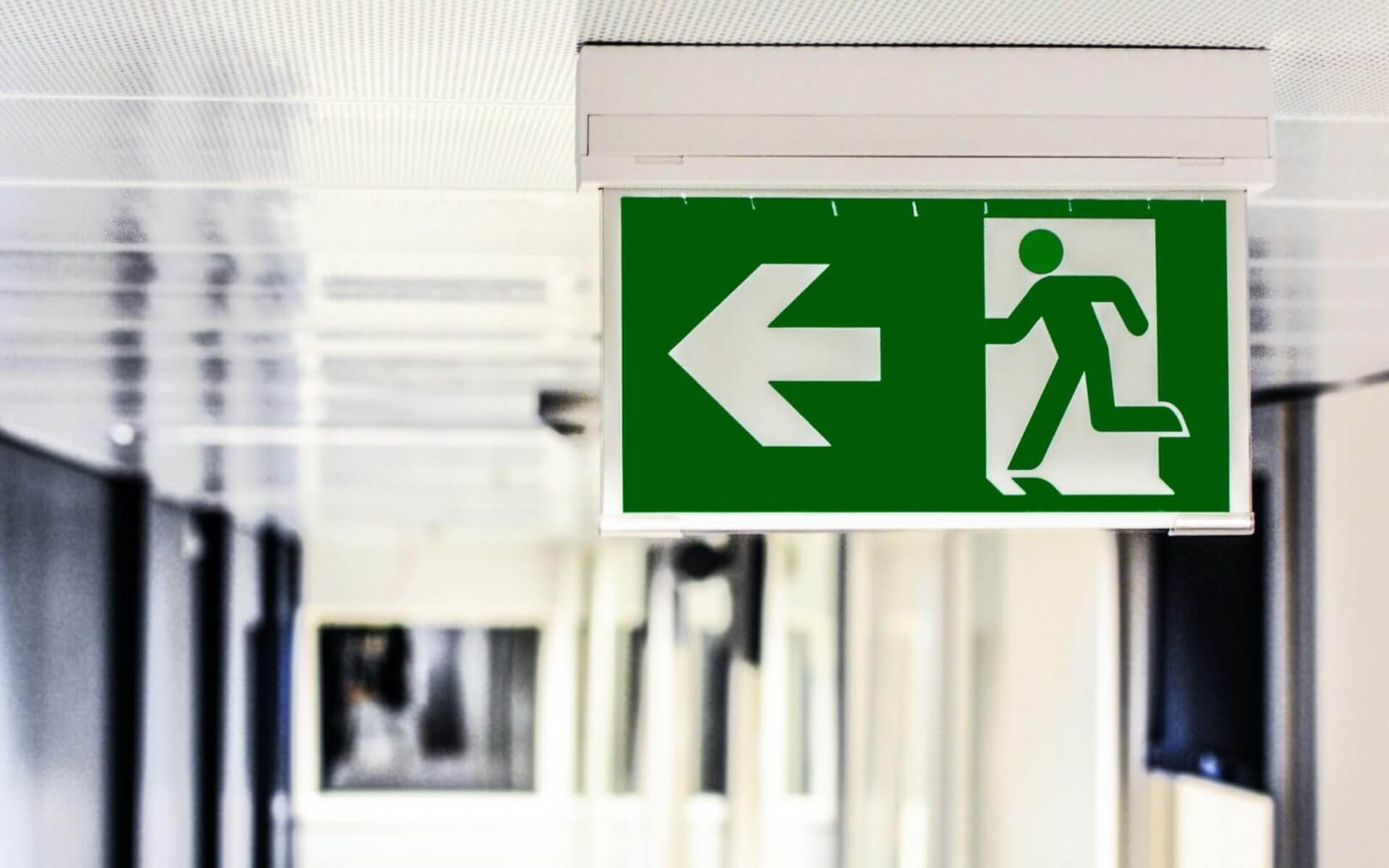
Typescript Access Modifiers, Type Safety And Return Types And Why They Are Important
November 15, 2021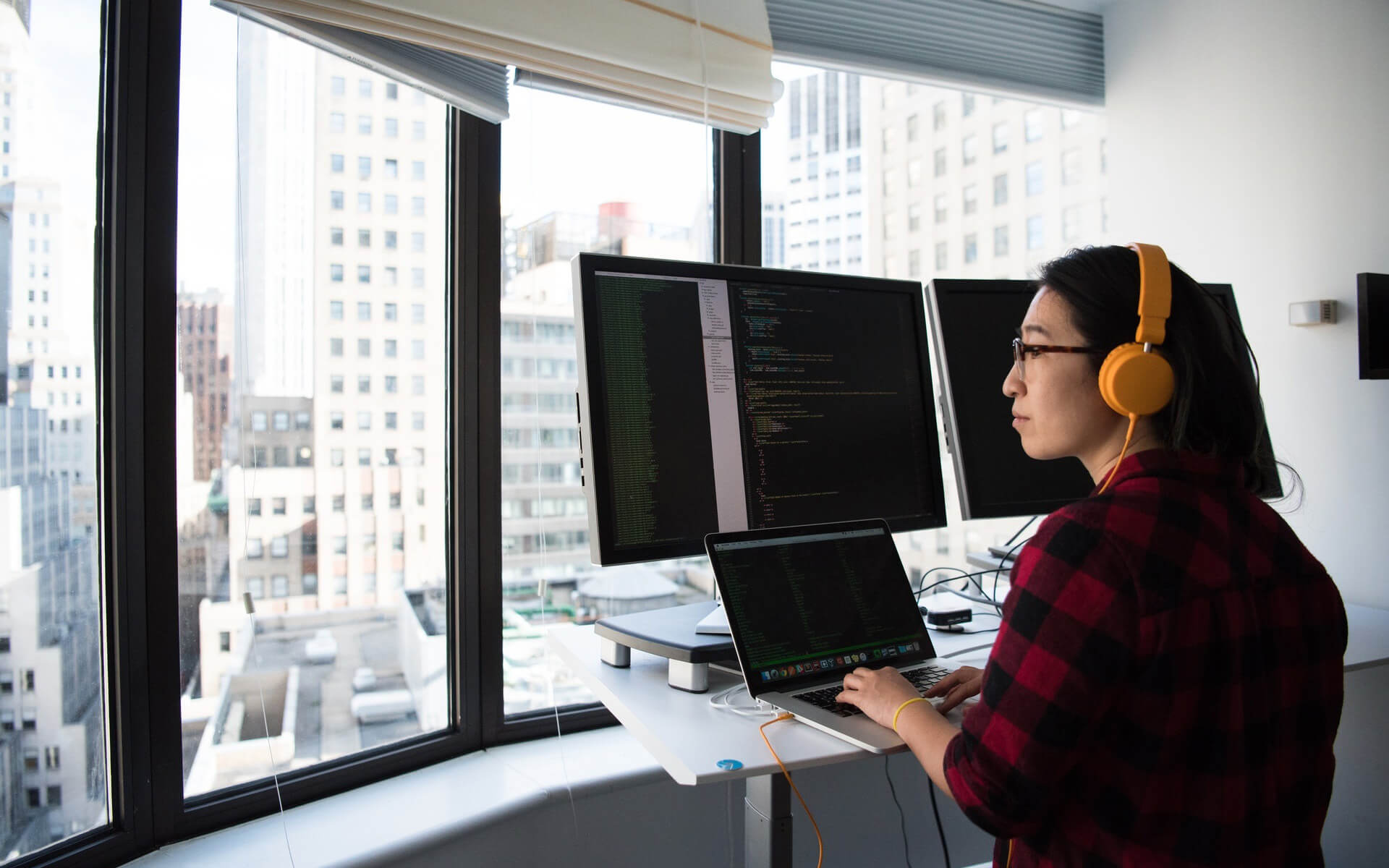
The past two weeks I was struggling to implement sockets for an Ionic Vue app that has a Laravel API. I also struggled finding documentation / tutorials that fit my specific needs. I finally found a solution and thought its the perfect thing to write a blog on to help anyone else struggling with this.
How To:
First lets set up the server side:
In your Laravel .env file we will need to configure the driver. The driver we will be using is Redis. The config settings you will need is as follows:
BROADCAST_DRIVER=redis CACHE_DRIVER=file QUEUE_CONNECTION=sync SESSION_DRIVER=file SESSION_LIFETIME=120 REDIS_PASSWORD=null REDIS_PORT=6379
Now we will need to install Redis. This is how to install Redis on a Windows machine:
- Go to this link.
- Download the .msi file and install it.
Next we will need to setup and install Laravel Echo Server
To install run the below in your terminal:
npm install -g laravel-echo-server
Next run the following in order to initialize and setup the server:
laravel-echo-server init
You will be presented with the following questions:
Do you want to run this server in development mode? Yes Which port would you like to serve from? 6001 Which database would you like to use to store presence channel members? redis Enter the host of your Laravel authentication server. your-domain.test Will you be serving on http or https? http Do you want to generate a client ID/Key for HTTP API? No Do you want to setup cross domain access to the API? Yes Specify the URI that may access the API: * Enter the HTTP methods that are allowed for CORS: GET, POST Enter the HTTP headers that are allowed for CORS: Origin, Content-Type, X-Auth-Token, X-Requested-With, Accept, Authorization, X-CSRF-TOKEN, X-Socket-Id What do you want this config to be saved as? laravel-echo-server.json
Next we will need to make a broadcast for the client side to listen to. You can run the below to create a broadcast:
php artisan make:event Example
In app/Events locate the new event that has been generated and update the following:
- On line 13 add implements ShouldBroadcast
- On line 34 change PrivateChannel to Channel
To run your Laravel Echo Server run the below in your terminal:
laravel-echo-server start
Next to setup the client side:
We will need to install Laravel Echo and Socket.io with the following command:
npm install laravel-echo-ionic socket.io-client
In your App.vue file add the below into the script tags, so that the ionic app always can connect to your Laravel Echo Server no mater what page the user is on.
import { Echo } from "laravel-echo-ionic"; window.io = require('socket.io-client'); window.Echo = new Echo({ broadcaster: "socket.io", host: "127.0.0.1:6001", }); window.Echo.connector.socket.on("connect", function () { console.log("CONNECTED"); }); window.Echo.connector.socket.o n("reconnecting", function () { console.log("CONNECTING"); }); window.Echo.connector.socket.o n("disconnect", function () { console.log("DISCONNECTED"); });
To listen to a broadcast we then use the below code on the page you want the app to be listening to a particular channel.
mounted() { window.Echo.channel("example"); window.Echo.connector.socket.on("App\\Events\\Example", (data) => { //add code that you want to trigger when this event occurs } },
Enjoy Coding!