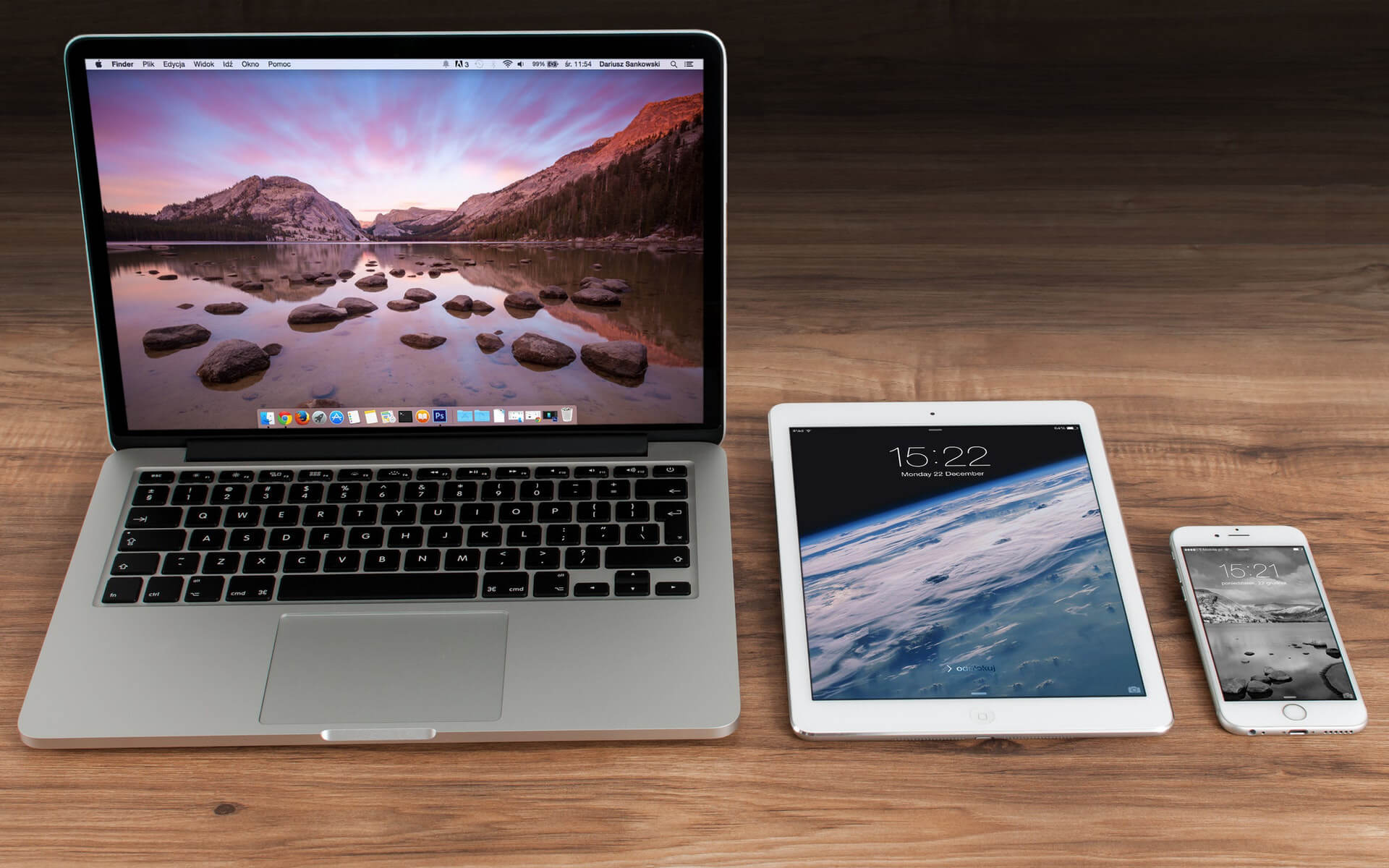
Difference Between Screen Size, Resolution And Display Size
September 8, 2020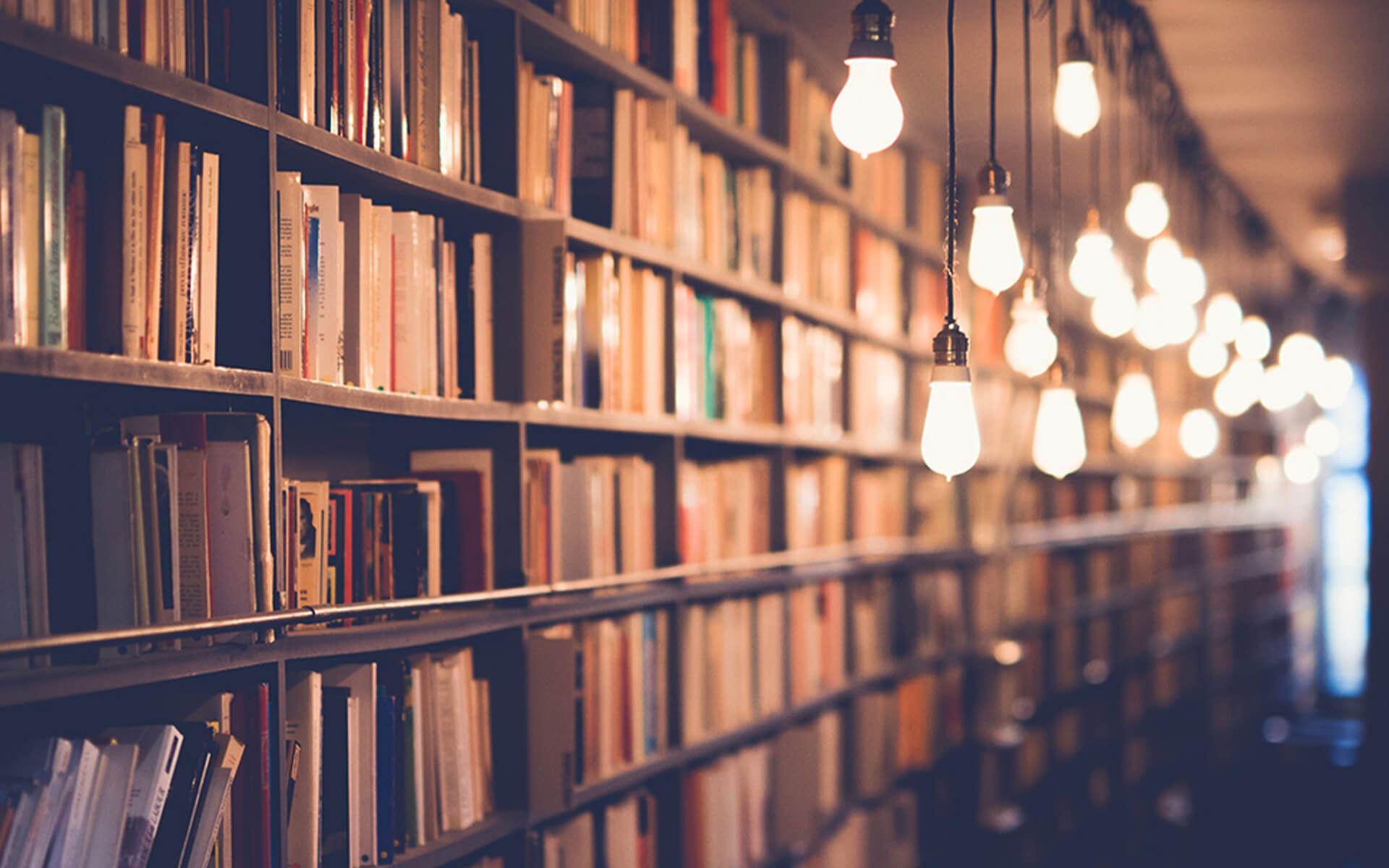
Upskilling Is The Key To Moving Forward
September 21, 2020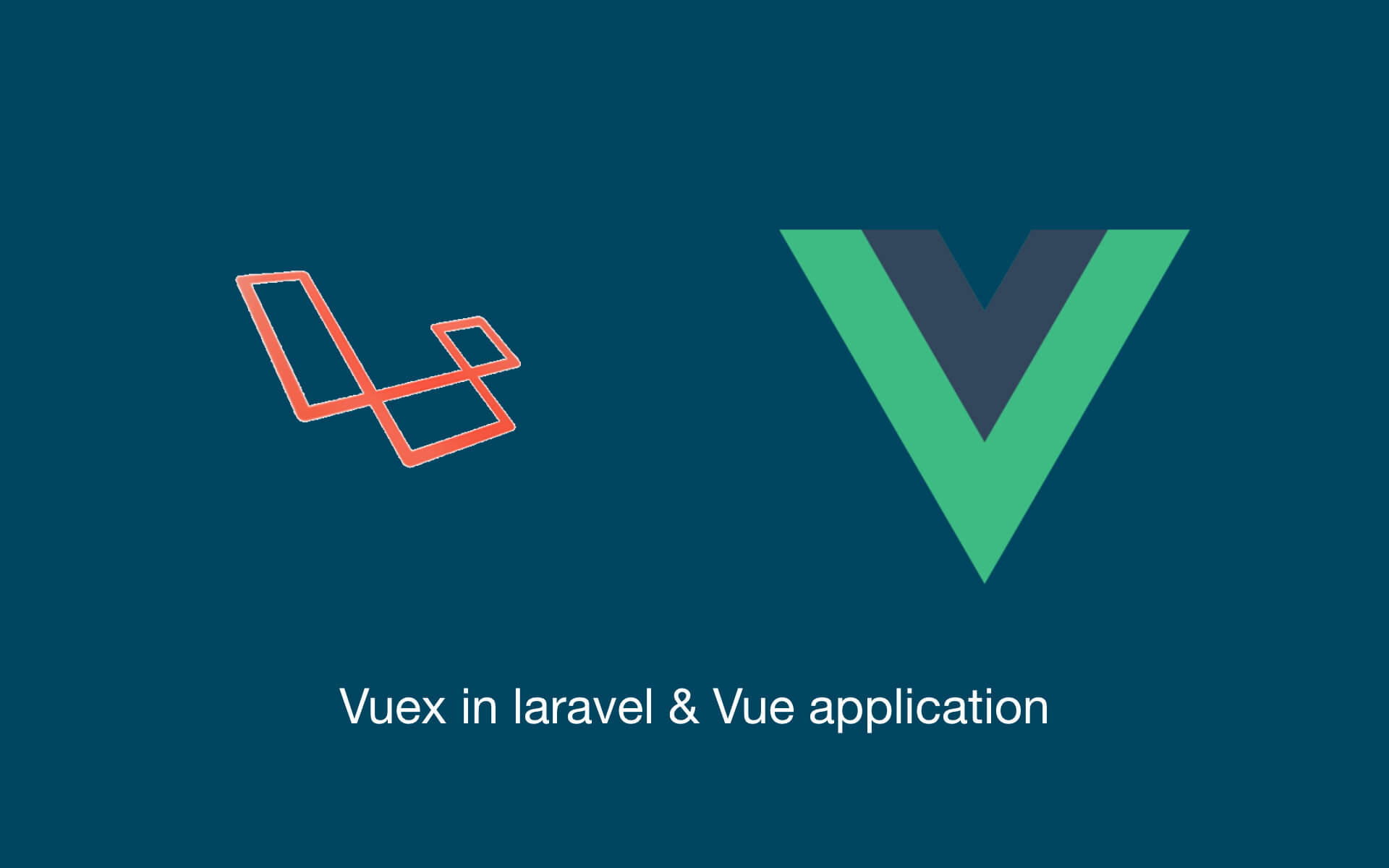
Firstly what is Vuex?
Well the text book definition would be:
Vuex is a state management pattern + library for Vue.js applications. It serves as a centralized store for all the components in an application, with rules ensuring that the state can only be mutated in a predictable fashion.
What does that mean?
Well every application has State which is information your program manipulates to accomplish some task. It is data or information that gets changed or manipulated throughout the runtime of a program, and vuex manages that state for you in a fashion thats easy to understand and is predictable.
Cool, but why should I use it?
Thats a good question, you could defiantly use emit or a central service to do the same thing, but you will realise quickly as your application grows, things become really messy fast and difficult to manage all at once, and Vuex takes care of all of that for you.
Great so we got that out the way lets look into setting it up.
Installation
start simply by navigating to your project directory in terminal or prompt, and inserting the following line.
npm install vuex --save
then import Vuex under your Vue import
Now We want to setup our store, where we will keep all of our mutators, actions, state, and getters, but wait what are those?
So state is a object of all the variables that we will parse around our application.
getters are methods that get information/data.
mutators are methods that mutate the state, in other words change data,
and actions are methods that do something, like an http request or can call a mutation asynchronously.
so this is what our store can look like
You will notice we have a property thats called modules, and this is an object where we can modularise a section to a specific component, such as AddToCart or ShoppingList.
Now import this store and use it in the Vue instance.
Now we can make our state, getters, mutators and actions objects.
state:
getters:
mutators:
actions:
And there you go vuex is setup now we can just use it thoughtout our application by simply going into a component, make a computed method and called the store with
computed:{
getUser(){
return this.$store.getters.getUser
}
},
methods:{
addUser(username){
this.$store.dispatch('addUser', username:username);
}
}
Then just call it in the template file with
{{getUser}}