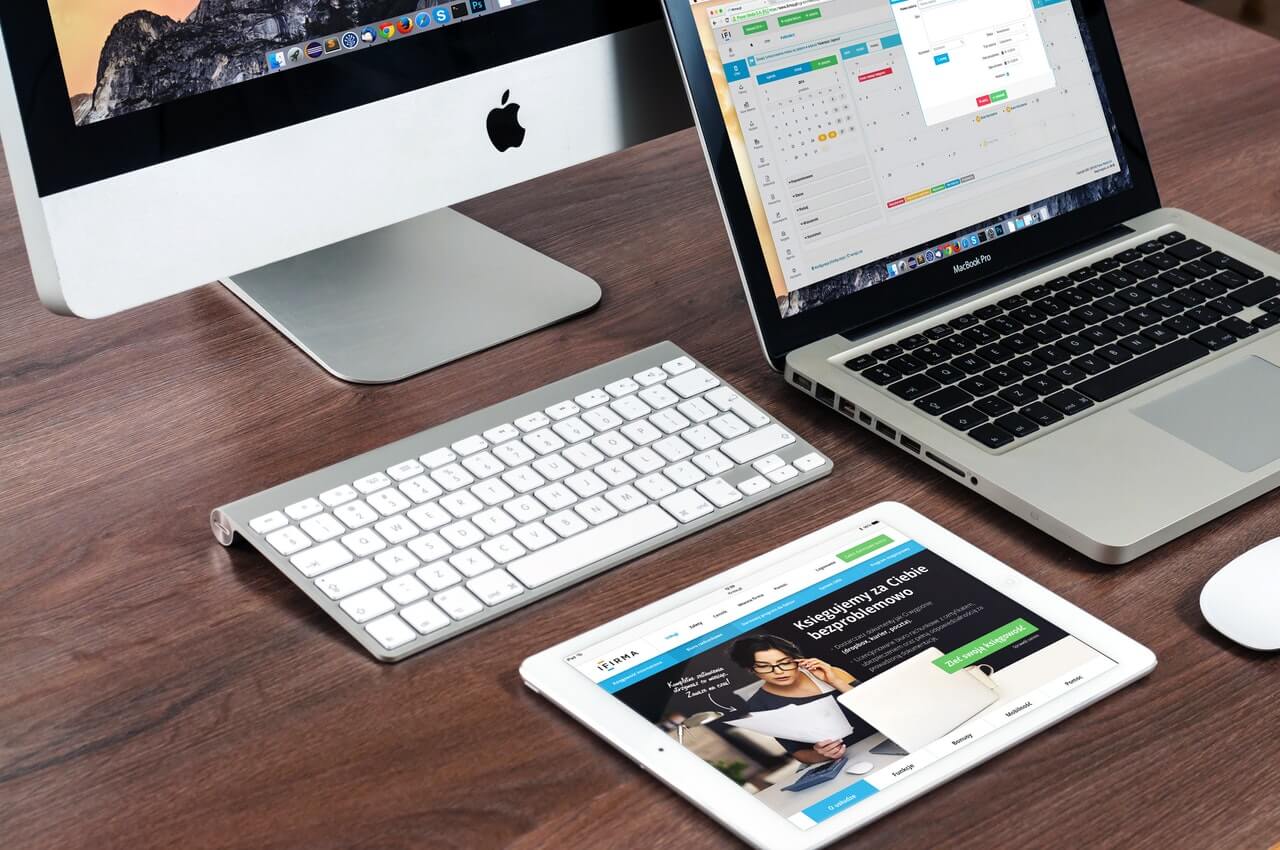
The Importance Of Website Maintenance
July 2, 2019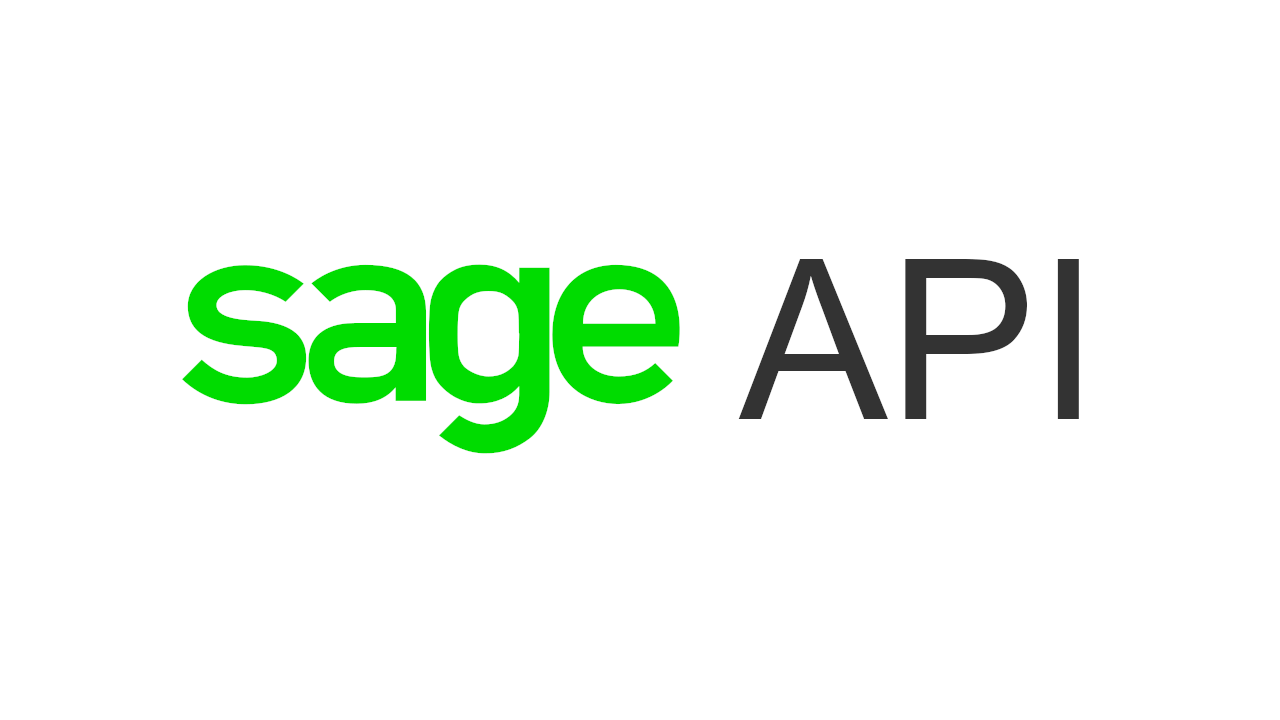
Getting Your Sage One Company ID For API Integration
September 6, 2019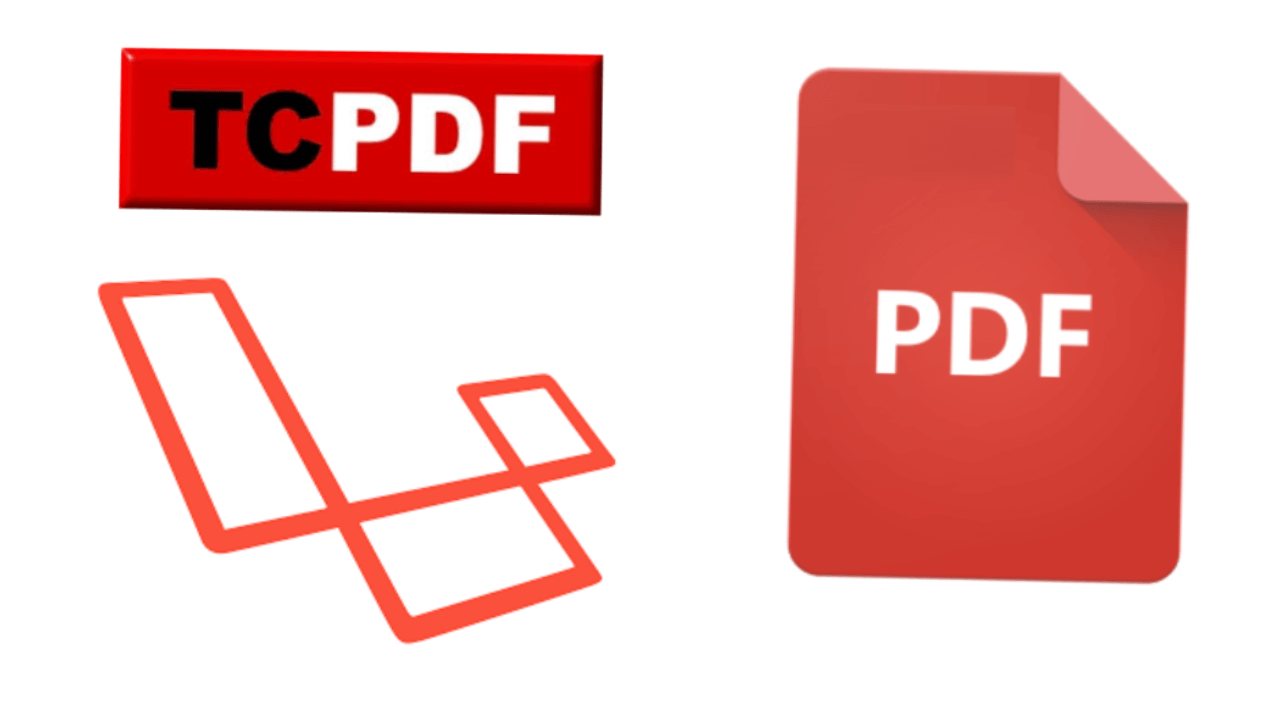
There are many reasons to digitally sign a PDF. The main 2 being to prove that a PDF hasn’t been edited and to prove that you are the author of the said PDF. You’re going to need 2 things for this tutorial. A certificate (e.g. alice.crt) and a private key (e.g. alice.key). I have provided some example certificates.
Download Example Certificates.
To generate PDFs, we can use TCPDF. TCPDF is a free, open source PHP class for generating PDFs. You can manually import TCPDF into any PHP project, but to make our lives easier there is a free Laravel service provider that will allow us to easily use TCPDF in any Laravel project. You can read more about the Laravel service provider here.
Generating the PDF
First lets begin by generating installing tcpdf-laravel.
composer require elibyy/tcpdf-laravel
If you don’t use auto-discovery, then add the following to your ‘config/app.php’.
'providers' => [ //... Elibyy\TCPDF\ServiceProvider::class, ] //... 'aliases' => [ //... 'PDF' => Elibyy\TCPDF\Facades\TCPDF::class ]
Now import tcpdf-laravel into your project wherever you wish to use it:
use Elibyy\TCPDF\Facades\TCPDF;
You can now use tcpdf to generate PDFs. We will do this using Laravels views. What we are doing is creating HTML code and then using TCPDF to render that into a PDF.
$view = view('pdf', [ 'content' = 'Lorem ipsum dolor' ]); $html = $view->render(); $pdf = new TCPDF(); $pdf::AddPage(); $pdf::writeHTML($html); $pdf_path = env('STORAGE_PATH') . 'https://lavalamplab.b-cdn.net/pdf_name.pdf'; $pdf_out = $pdf::Output($pdf_path, 'F');
The above code renders the pdf.blade.html file into $html
and then uses tcpdf to generate it into a pdf and store it somewhere on disk. You can just output the PDF to the client by replacing $pdf_out = $pdf::Output($pdf_path, 'F');
with $pdf::Output();
Now we can do the same thing and also digitally sign the PDF in the process.
$certificate = 'file://'. realpath('../storage/cert/alice.crt'); $private_key = 'file://'. realpath('../storage/cert/alice.key'); $view = view('pdf', [ 'content' = 'Lorem ipsum dolor' ]); $html = $view->render(); $pdf = new TCPDF(); $pdf::AddPage(); $pdf::writeHTML($html); // set additional information $info = array( 'Name' => 'Name of PDF', 'Location' => '', 'Reason' => 'Proof of author', 'ContactInfo' => 'info@example.co.za', ); // set document signature $pdf::setSignature($certificate, $private_key, '', '', 2, $info); $pdf_path = env('STORAGE_PATH').'https://lavalamplab.b-cdn.net/files/pdf_name.pdf'; $pdf_out = $pdf::Output($pdf_path, 'F');
You need to use the realpath()
function to get the path to the key and certificate.
If you require more information about TCPDF I highly recommend these 2 resources:
3 Comments
Hi, it’s works for me. thank so much!
You should avoid using env() helper outside config! It will break your app when you cache the config.
I need to sign the same document with two different certificates. Can you help me?